vue2 封装简单的表格
时间: 2023-07-18 09:04:45 浏览: 226
可以基于 Element UI 的 Table 组件进行封装,代码如下:
```vue
<template>
<el-table :data="tableData" :height="height" :max-height="maxHeight" :stripe="stripe" :border="border"
:show-header="showHeader" :highlight-current-row="highlightCurrentRow" :row-class-name="rowClassName"
:row-style="rowStyle" :cell-class-name="cellClassName" :cell-style="cellStyle" :header-row-class-name="headerRowClassName"
:header-row-style="headerRowStyle" :header-cell-class-name="headerCellClassName" :header-cell-style="headerCellStyle"
:row-key="rowKey" :empty-text="emptyText" :default-expand-all="defaultExpandAll" :expand-row-keys="expandRowKeys"
:default-sort="defaultSort" :tooltip-effect="tooltipEffect" :span-method="spanMethod" :select-on-indeterminate="selectOnIndeterminate"
:indent="indent" :lazy="lazy" :load="load" :tree-props="treeProps" :frozen-columns="frozenColumns"
:frozen-right-columns="frozenRightColumns" :edit-config="editConfig" :edit-rules="editRules" :show-summary="showSummary"
:sum-text="sumText" :summary-method="summaryMethod" :scrollable="scrollable" :resizable="resizable"
:size="size" :fit="fit" :render-content="renderContent" :row-gutter="rowGutter">
<slot></slot>
</el-table>
</template>
<script>
export default {
name: 'MyTable',
props: {
tableData: {
type: Array,
default: () => []
},
height: {
type: String,
default: ''
},
maxHeight: {
type: String,
default: ''
},
stripe: {
type: Boolean,
default: true
},
border: {
type: Boolean,
default: true
},
showHeader: {
type: Boolean,
default: true
},
highlightCurrentRow: {
type: Boolean,
default: false
},
rowClassName: {
type: Function,
default: () => null
},
rowStyle: {
type: [Function, Object],
default: () => null
},
cellClassName: {
type: Function,
default: () => null
},
cellStyle: {
type: [Function, Object],
default: () => null
},
headerRowClassName: {
type: Function,
default: () => null
},
headerRowStyle: {
type: [Function, Object],
default: () => null
},
headerCellClassName: {
type: Function,
default: () => null
},
headerCellStyle: {
type: [Function, Object],
default: () => null
},
rowKey: {
type: [Function, String],
default: () => null
},
emptyText: {
type: String,
default: '暂无数据'
},
defaultExpandAll: {
type: Boolean,
default: false
},
expandRowKeys: {
type: Array,
default: () => []
},
defaultSort: {
type: Object,
default: () => null
},
tooltipEffect: {
type: String,
default: 'dark'
},
spanMethod: {
type: Function,
default: () => null
},
selectOnIndeterminate: {
type: Boolean,
default: true
},
indent: {
type: Number,
default: 16
},
lazy: {
type: Boolean,
default: false
},
load: {
type: Function,
default: () => null
},
treeProps: {
type: Object,
default: () => ({
hasChildren: 'hasChildren',
children: 'children'
})
},
frozenColumns: {
type: Array,
default: () => []
},
frozenRightColumns: {
type: Array,
default: () => []
},
editConfig: {
type: Object,
default: () => null
},
editRules: {
type: Object,
default: () => null
},
showSummary: {
type: Boolean,
default: false
},
sumText: {
type: String,
default: '合计'
},
summaryMethod: {
type: Function,
default: () => null
},
scrollable: {
type: Boolean,
default: true
},
resizable: {
type: Boolean,
default: true
},
size: {
type: String,
default: ''
},
fit: {
type: Boolean,
default: true
},
renderContent: {
type: Function,
default: () => null
},
rowGutter: {
type: Number,
default: 0
}
}
}
</script>
```
这里我们将所有的 Table 属性都作为 MyTable 的 props,通过 `<slot></slot>` 将所有的 Table 列表项插入到 MyTable 中,这样就可以实现一个简单的 Table 封装组件了。使用时只需要在父组件中传入数据和需要的属性即可。
例如:
```vue
<template>
<my-table :table-data="tableData" :stripe="false">
<el-table-column prop="date" label="日期"></el-table-column>
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="address" label="地址"></el-table-column>
</my-table>
</template>
<script>
import MyTable from './MyTable.vue'
export default {
components: {
MyTable
},
data() {
return {
tableData: [{
date: '2021-09-01',
name: '张三',
address: '北京市朝阳区'
}, {
date: '2021-09-02',
name: '李四',
address: '上海市浦东新区'
}]
}
}
}
</script>
```
阅读全文
相关推荐
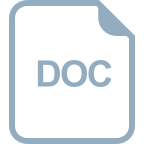
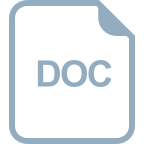
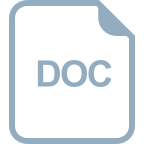
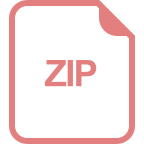
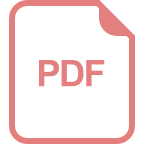
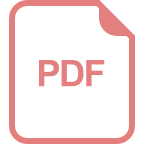











