用forkjoin 结合randomAccessfile 对一个文件分任务的复制
时间: 2024-06-11 22:08:35 浏览: 14
这里提供一种使用ForkJoin框架和RandomAccessFile实现对大文件的分任务复制的方法。
1. 首先,我们需要创建一个ForkJoin任务类,用于实现对文件的分任务复制。此任务类需要继承ForkJoinTask类,并实现compute方法。具体实现如下:
```java
public class FileCopyTask extends ForkJoinTask<Void> {
private static final int THRESHOLD = 1024 * 1024; // 每个任务最多复制 1MB
private String srcPath; // 源文件路径
private String dstPath; // 目标文件路径
private long start; // 当前任务开始复制的位置
private long end; // 当前任务结束复制的位置
public FileCopyTask(String srcPath, String dstPath, long start, long end) {
this.srcPath = srcPath;
this.dstPath = dstPath;
this.start = start;
this.end = end;
}
@Override
public Void compute() {
try (RandomAccessFile srcFile = new RandomAccessFile(srcPath, "r");
RandomAccessFile dstFile = new RandomAccessFile(dstPath, "rw")) {
srcFile.seek(start);
dstFile.seek(start);
byte[] buffer = new byte[THRESHOLD];
long remaining = end - start;
while (remaining > 0) {
int n = srcFile.read(buffer, 0, (int) Math.min(buffer.length, remaining));
if (n <= 0) {
break;
}
dstFile.write(buffer, 0, n);
remaining -= n;
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
@Override
public boolean exec() {
compute();
return true;
}
}
```
2. 接下来,我们需要创建一个ForkJoinPool,并提交任务到该池中。具体实现如下:
```java
public class FileCopy {
public static void copy(String srcPath, String dstPath) throws IOException {
long fileSize = new File(srcPath).length();
ForkJoinPool pool = new ForkJoinPool();
FileCopyTask task = new FileCopyTask(srcPath, dstPath, 0, fileSize);
pool.submit(task);
task.join(); // 等待任务完成
pool.shutdown(); // 关闭线程池
}
}
```
3. 最后,我们可以调用FileCopy.copy方法,实现对文件的分任务复制。具体使用方法如下:
```java
public static void main(String[] args) throws IOException {
FileCopy.copy("src.txt", "dst.txt");
}
```
需要注意的是,在实际使用中,我们需要根据文件大小和计算机的性能,合理设置每个任务的大小和线程池的大小,以达到最优的复制速度。
相关推荐
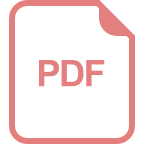
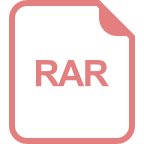
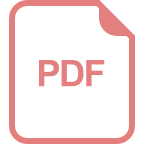
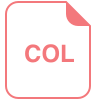













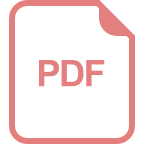