图片归一化代码python
时间: 2023-05-08 12:00:50 浏览: 114
图片归一化是一种处理图像的方法,它将原始图像的像素值缩放到0和1之间,以避免像素值过大或者过小导致数据异常的情况。以下是Python代码实现图像归一化:
```python
import cv2
import numpy as np
def normalize(image):
# 将图像像素值归一化到0和1之间
image -= np.min(image)
image /= np.max(image)
return image
# 读取图像数据
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 图像归一化
norm_img = normalize(img)
```
代码中首先用OpenCV库读取图片,并将其转为灰度图,然后定义了一个`normalize`函数,该函数将图像像素值减去最小值,然后再除以最大值,从而将像素缩放到0和1之间。最后,对读取的图像数据调用`normalize`函数,得到了归一化后的图像数据`norm_img`。这样做可以避免像素值过大或者过小,使得处理结果更加准确可靠。
相关问题
json转txt格式,X1,Y1图片中心坐标宽高 ,图片大小不同并归一化,python代码
以下是将JSON转换为TXT格式,并将坐标转换为图片中心坐标和宽高的Python代码示例:
```python
import json
import csv
def json_to_txt(json_file, txt_file):
with open(json_file, 'r') as f:
data = json.load(f)
with open(txt_file, 'w', newline='') as f:
writer = csv.writer(f, delimiter=',')
writer.writerow(['X', 'Y', 'Width', 'Height'])
for obj in data['objects']:
x1 = obj['x1']
y1 = obj['y1']
x2 = obj['x2']
y2 = obj['y2']
# 计算中心坐标
width = x2 - x1
height = y2 - y1
x = (x1 + x2) / 2
y = (y1 + y2) / 2
# 归一化处理
x /= data['width']
y /= data['height']
width /= data['width']
height /= data['height']
writer.writerow([x, y, width, height])
# 使用示例
json_file = 'input.json'
txt_file = 'output.txt'
json_to_txt(json_file, txt_file)
```
在上述代码中,我们首先读取JSON文件并加载数据。然后,我们按照给定的逻辑计算目标框的中心坐标和宽高。接下来,我们将这些值进行归一化处理,通过将它们除以图像的宽度和高度来实现。最后,我们将结果写入到TXT文件中。请确保将实际的JSON文件名和输出的TXT文件名替换为你自己的文件名。
批量json转txt格式,X1,Y1图片中心坐标宽高 ,图片大小不同并归一化,python代码
以下是将批量JSON文件转换为TXT格式,并将坐标转换为图片中心坐标和宽高的Python代码示例:
```python
import json
import csv
import os
def json_to_txt(json_folder, txt_folder):
if not os.path.exists(txt_folder):
os.makedirs(txt_folder)
json_files = os.listdir(json_folder)
for json_file in json_files:
if not json_file.endswith('.json'):
continue
json_path = os.path.join(json_folder, json_file)
txt_file = json_file.replace('.json', '.txt')
txt_path = os.path.join(txt_folder, txt_file)
with open(json_path, 'r') as f:
data = json.load(f)
with open(txt_path, 'w', newline='') as f:
writer = csv.writer(f, delimiter=',')
writer.writerow(['X', 'Y', 'Width', 'Height'])
for obj in data['objects']:
x1 = obj['x1']
y1 = obj['y1']
x2 = obj['x2']
y2 = obj['y2']
# 计算中心坐标
width = x2 - x1
height = y2 - y1
x = (x1 + x2) / 2
y = (y1 + y2) / 2
# 归一化处理
x /= data['width']
y /= data['height']
width /= data['width']
height /= data['height']
writer.writerow([x, y, width, height])
# 使用示例
json_folder = 'input_folder'
txt_folder = 'output_folder'
json_to_txt(json_folder, txt_folder)
```
在上述代码中,我们首先创建一个输出文件夹(如果不存在)。然后,我们列出输入文件夹中的所有JSON文件。对于每个JSON文件,我们按照之前的逻辑进行处理,并将结果写入到对应的TXT文件中。请确保将实际的输入文件夹和输出文件夹路径替换为你自己的文件夹路径。
阅读全文
相关推荐







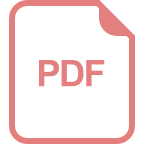





