from math import sqrt for n in range(99, 81, -1): root = sqrt(n) if root == int(root): print(n) break else: print("Didn't find it!") root起什么作用?if root == int(root):这句话是什么意思
时间: 2024-04-05 17:36:07 浏览: 207
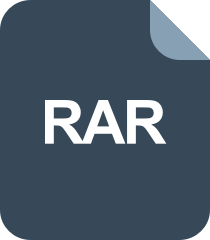
sqrt_LUT8.rar_ROOT_s-lut_sqrt_sqrt sqrt lut_square root vhdl
在这段代码中,`root` 变量的作用是用于存储当前数字 `n` 的平方根。`sqrt(n)` 函数是 Python 内置的求平方根的函数,它返回一个浮点数。
在 `if root == int(root):` 这句话中,`int(root)` 的作用是将平方根 `root` 转换为整数。这是因为如果 `n` 是完全平方数,那么它的平方根必然是整数,而如果 `n` 不是完全平方数,那么它的平方根就不是整数。因此,`if root == int(root):` 的作用是判断当前数字 `n` 的平方根 `root` 是否为整数,如果是,则说明 `n` 是完全平方数,程序就会输出 `n` 并结束循环。如果不是,则继续循环,直到找到一个完全平方数或者遍历完整个区间。
阅读全文
相关推荐
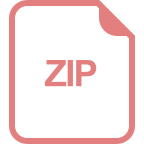
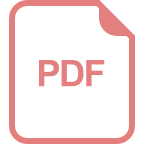
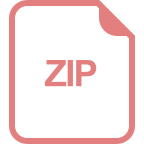
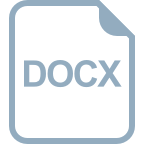
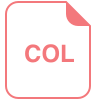
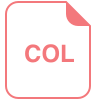
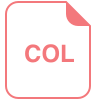
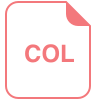
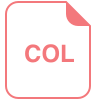
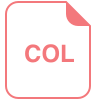
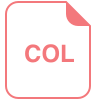
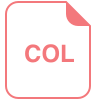
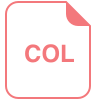



