【深入剖析sqrt函数:揭秘平方根计算的数学奥秘和实用技巧】
发布时间: 2024-07-12 20:03:57 阅读量: 160 订阅数: 29 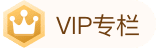
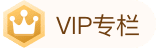
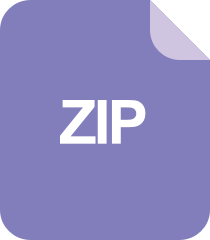
bigtab.zip
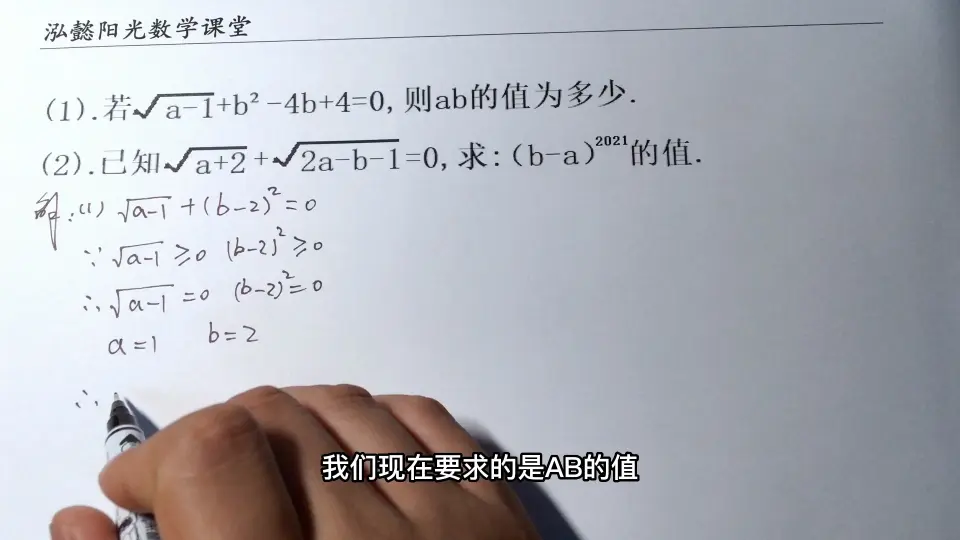
# 1. 平方根的数学基础
平方根是数学中一个基本概念,表示一个数的正数乘以自身所得的结果。对于非负实数 x,其平方根记为 √x。
### 1.1 平方根的定义
平方根的定义如下:
```
对于非负实数 x,其平方根 √x 是满足以下方程的唯一正实数:
√x * √x = x
```
### 1.2 平方根的性质
平方根具有以下性质:
- **非负性:**对于非负实数 x,其平方根 √x 始终是非负的。
- **唯一性:**对于非负实数 x,其平方根 √x 唯一存在。
- **乘法性:**对于非负实数 x 和 y,有 √(xy) = √x * √y。
- **除法性:**对于非负实数 x 和 y,有 √(x/y) = √x / √y。
# 2. Sqrt函数的实现原理
### 2.1 牛顿迭代法
#### 2.1.1 迭代公式的推导
牛顿迭代法是一种求解方程根的迭代算法,其核心思想是通过不断逼近根值来求解方程。对于平方根计算,其方程为:
```
x^2 - a = 0
```
其中,`a` 为非负实数。
牛顿迭代法的迭代公式为:
```
x_{n+1} = x_n - f(x_n) / f'(x_n)
```
其中,`x_n` 为第 `n` 次迭代的近似值,`f(x)` 为方程,`f'(x)` 为方程的导数。
对于平方根计算,其迭代公式变为:
```
x_{n+1} = x_n - (x_n^2 - a) / (2x_n)
```
#### 2.1.2 算法的收敛性和复杂度
牛顿迭代法具有二次收敛性,这意味着每次迭代后,近似值的精度会平方倍地提高。
算法的复杂度为 `O(log(ε))`,其中 `ε` 为所需的精度。
### 2.2 二分法
#### 2.2.1 二分查找的原理
二分法是一种在有序数组中查找元素的算法。其原理是将数组分成两半,并根据目标元素与中间元素的关系,确定目标元素位于前半部分还是后半部分,然后重复此过程,直到找到目标元素或确定目标元素不存在。
#### 2.2.2 平方根计算的二分实现
对于平方根计算,二分法的实现如下:
```python
def sqrt_binary_search(a, epsilon):
"""
使用二分法计算平方根。
参数:
a: 非负实数,要计算平方根的数。
epsilon: 允许的误差范围。
返回:
a 的平方根的近似值。
"""
low = 0
high = a
while high - low > epsilon:
mid = (low + high) / 2
if mid * mid < a:
low = mid
else:
high = mid
return low
```
该算法的复杂度为 `O(log(a))`。
#### 代码块逻辑分析
```python
def sqrt_binary_search(a, epsilon):
"""
使用二分法计算平方根。
参数:
a: 非负实数,要计算平方根的数。
epsilon: 允许的误差范围。
返回:
a 的平方根的近似值。
"""
# 初始化搜索范围
low = 0
high = a
# 迭代查找平方根
while high - low > epsilon:
# 计算中间值
mid = (low + high) / 2
# 判断中间值是否符合条件
if mid * mid < a:
# 中间值太小,更新下界
low = mid
else:
# 中间值太大,更新上界
high = mid
# 返回平方根的近似值
return low
```
#### 参数说明
* `a`: 要计算平方根的非负实数。
* `epsilon`: 允许的误差范围,用于控制迭代停止的条件。
#### 逻辑分析
该算法使用二分法来查找 `a` 的平方根。它首先将搜索范围初始化为 `[0, a]`,然后不断地将搜索范围缩小,直到满足以下条件:
* `high - low <= epsilon`:表示搜索范围已经足够小,可以认为已经找到了平方根的近似值。
在每次迭代中,算法计算搜索范围的中间值 `mid`,并检查 `mid * mid` 是否等于 `a`。如果等于,则 `mid` 就是平方根的近似值,算法直接返回 `mid`。如果小于,则表示平方根大于 `mid`,算法将 `low` 更新为 `mid`。如果大于,则表示平方根小于 `mid`,算法将 `high` 更新为 `mid`。
通过不断地缩小搜索范围,算法最终会找到一个近似值,使得 `mid * mid` 与 `a` 的差值小于或等于 `epsilon`。
# 3. Sqrt函数的应用技巧
### 3.1 优化平方根计算的精度
#### 3.1.1 迭代次数的选取
牛顿迭代法的收敛速度与迭代次数有关。迭代次数越多,精度越高,但计算量也越大。一般情况下,迭代 5-10 次即可获得较高的精度。
#### 3.1.2 初始估计值的优化
牛顿迭代法的收敛速度还与初始估计值有关。初始估计值越接近平方根的真实值,收敛速度越快。可以利用一些近似方法来获得一个较好的初始估计值,例如:
```python
def initial_estimate(x):
"""
获取x的平方根的初始估计值
"""
return x / 2
```
### 3.2 平方根的近似计算
在某些情况下,精确的平方根计算并不是必需的,近似计算可以满足需求。以下介绍两种近似计算平方根的方法:
#### 3.2.1 泰勒级数展开
泰勒级数展开可以将平方根函数近似为多项式。对于x > 0,平方根函数的泰勒级数展开式为:
```
sqrt(x) ≈ 1 + 1/2 * x - 1/8 * x^2 + 1/16 * x^3 - 5/128 * x^4 + ...
```
截断到前n项,可以得到平方根的近似值:
```python
def taylor_sqrt(x, n):
"""
泰勒级数展开近似计算平方根
"""
result = 1
for i in range(1, n + 1):
result += (1 / (2 ** i)) * (x ** i)
return result
```
#### 3.2.2 分段线性逼近
分段线性逼近将平方根函数划分为多个线性段,在每个线性段内使用线性函数近似平方根函数。
```python
def linear_sqrt(x, segments):
"""
分段线性逼近近似计算平方根
"""
segment_size = 1 / segments
segment_index = int(x / segment_size)
start = segment_index * segment_size
end = (segment_index + 1) * segment_size
slope = (end - start) / (end ** 2 - start ** 2)
y_intercept = start - slope * start ** 2
return slope * x + y_intercept
```
# 4. Sqrt函数的编程实践
### 4.1 不同编程语言中的Sqrt函数
不同的编程语言提供了不同的Sqrt函数实现,以满足不同的精度、性能和平台需求。
#### 4.1.1 C语言中的sqrt函数
C语言中提供了标准库函数`sqrt()`来计算平方根。其原型为:
```c
double sqrt(double x);
```
其中,`x`为要计算平方根的非负实数。该函数返回`x`的平方根,如果`x`为负数,则返回`NAN`(非数字)。
#### 4.1.2 Python中的math.sqrt函数
Python中提供了`math`模块,其中包含`sqrt()`函数来计算平方根。其原型为:
```python
math.sqrt(x)
```
其中,`x`为要计算平方根的非负实数。该函数返回`x`的平方根,如果`x`为负数,则引发`ValueError`异常。
### 4.2 平方根计算的性能优化
在某些应用场景中,平方根计算的性能至关重要。以下是一些常见的优化技术:
#### 4.2.1 缓存机制的应用
对于重复计算相同的平方根值的情况,可以利用缓存机制来避免重复计算。例如,在图像处理中,经常需要计算大量像素点的平方根。通过将计算过的平方根值存储在缓存中,可以显著提高计算效率。
#### 4.2.2 SIMD指令集的利用
SIMD(单指令多数据)指令集可以并行处理多个数据元素。现代处理器通常支持SIMD指令集,如SSE(流式SIMD扩展)和AVX(高级矢量扩展)。利用SIMD指令集可以显著提高平方根计算的性能,特别是在处理大量数据时。
### 代码示例
以下是一个使用C语言实现Sqrt函数的代码示例:
```c
#include <math.h>
double sqrt(double x) {
if (x < 0) {
return NAN;
}
double guess = x / 2;
while (fabs(guess * guess - x) > 0.00001) {
guess = (guess + x / guess) / 2;
}
return guess;
}
```
该代码使用牛顿迭代法来计算平方根。`fabs()`函数计算绝对值。
以下是一个使用Python中的`math.sqrt()`函数计算平方根的代码示例:
```python
import math
x = 10
result = math.sqrt(x)
print(result) # 输出:3.1622776601683795
```
在实际应用中,根据具体需求选择合适的Sqrt函数实现和优化技术,可以有效提高平方根计算的效率和精度。
# 5.1 图像处理中的平方根变换
### 5.1.1 图像增强
在图像处理中,平方根变换是一种常用的图像增强技术,它可以提高图像的对比度,增强图像中的细节。平方根变换的原理是将图像中每个像素的灰度值进行平方根运算,从而将图像的灰度分布拉伸。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 应用平方根变换
sqrt_image = np.sqrt(image)
# 显示原图和平方根变换后的图像
cv2.imshow('Original Image', image)
cv2.imshow('Square Root Transformed Image', sqrt_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 5.1.2 特征提取
平方根变换还可用于图像特征提取。通过对图像进行平方根变换,可以提取图像中具有特定纹理和模式的区域。这些区域在平方根变换后的图像中会表现出更强的对比度,便于后续的特征识别和分类。
```python
import cv2
import numpy as np
# 读取图像
image = cv2.imread('image.jpg')
# 应用平方根变换
sqrt_image = np.sqrt(image)
# 使用Sobel算子提取边缘
edges = cv2.Sobel(sqrt_image, cv2.CV_64F, 1, 0, ksize=5)
# 显示原图、平方根变换后的图像和边缘提取结果
cv2.imshow('Original Image', image)
cv2.imshow('Square Root Transformed Image', sqrt_image)
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
0
0
相关推荐
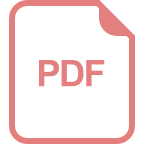
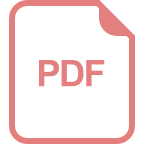
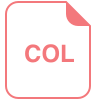
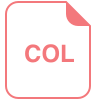
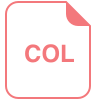
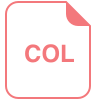
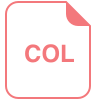
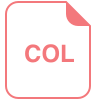