python去除英文标点符号
时间: 2023-09-24 09:06:20 浏览: 112
### 回答1:
您可以使用Python的string模块中的`punctuation`变量来去除英文标点符号。以下是示例代码:
```python
import string
# 示例文本
text = "Hello, World! This is a test sentence."
# 去除标点符号
text_without_punct = text.translate(str.maketrans("", "", string.punctuation))
print(text_without_punct)
```
输出结果:
```
Hello World This is a test sentence
```
在这个示例中,我们使用了`translate()`方法来去除标点符号,并使用了`str.maketrans()`方法来创建一个映射表,将标点符号映射为空字符。我们还使用了Python的string模块中的`punctuation`变量来获取所有英文标点符号的字符串。
### 回答2:
可以使用Python的re模块中的正则表达式来去除英文标点符号。
首先,导入re模块:
import re
然后,定义一个函数来去除英文标点符号:
def remove_punctuation(text):
# 定义正则表达式,匹配英文标点符号
pattern = r'[^\w\s]'
# 使用sub方法将匹配到的标点符号替换为空字符
no_punct = re.sub(pattern, '', text)
return no_punct
接下来,调用函数并传入待处理的文本:
text = "Hello, world!"
clean_text = remove_punctuation(text)
print(clean_text)
运行代码,将会输出去除了标点符号的文本:
Hello world
这样,就可以通过正则表达式和re模块来实现Python去除英文标点符号的功能了。
### 回答3:
在Python中,可以使用字符串的replace()方法来去除英文标点符号。
以下是一个简单的示例代码:
```python
def remove_punctuation(text):
punctuation = '''!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~'''
for char in punctuation:
text = text.replace(char, '')
return text
# 测试示例
sentence = "Hello, World!"
cleaned_sentence = remove_punctuation(sentence)
print(cleaned_sentence)
```
上述代码定义了一个remove_punctuation函数,它接受一个字符串作为参数。函数内部定义了一个包含所有英文标点符号的字符串punctuation。
接下来,我们使用for循环迭代punctuation中的每个字符,然后使用字符串的replace()方法将这些字符替换为空字符串。
最后,函数返回替换完成后的字符串。在上述示例中,我们将字符串"Hello, World!"传递给remove_punctuation函数,并将结果赋值给cleaned_sentence变量。在打印cleaned_sentence之后,我们将获得一个没有标点符号的字符串"Hello World"。
这是通过迭代字符串中的每个字符,并将其替换为空字符串来实现的。最终,我们得到了一个没有英文标点符号的字符串。
相关推荐
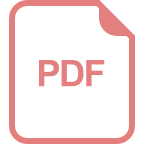
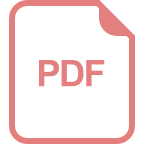














