python实现去除文件的标点符号和英文
时间: 2023-06-27 14:02:05 浏览: 168
可以使用Python的re模块和string模块来去除文件的标点符号和英文。
下面是一个示例代码:
```python
import re
import string
# 读取文件内容
with open('filename.txt', 'r') as f:
content = f.read()
# 去除标点符号和英文
content = re.sub('[%s]' % re.escape(string.punctuation + string.ascii_letters), '', content)
# 输出处理后的内容
print(content)
```
解释一下代码:
1. 使用`open`函数打开文件,并使用`read`方法读取文件内容。
2. 使用正则表达式去除标点符号和英文,`[%s]`表示匹配任何一个字符集中的字符,`re.escape`用来转义标点符号和英文字母。
3. 最后输出处理后的内容。
注意,这种方法只适用于英文文本,对于中文文本需要使用中文分词等方法来处理。
相关问题
python实现去除文件的标点符号
可以使用Python内置的string模块来实现。
示例代码如下:
```python
import string
with open("example.txt", "r") as f:
text = f.read()
# 去除标点符号
text = text.translate(str.maketrans("", "", string.punctuation))
print(text)
```
这段代码会读取一个名为`example.txt`的文本文件,并去除其中所有的标点符号。最后将处理后的文本打印出来。你可以根据需要修改文件名和路径。
python去除英文标点符号
可以使用Python内置的string库以及正则表达式来去除英文标点符号。
以下是一个示例代码:
```python
import string
import re
def remove_punctuation(text):
# 去除string库中的标点符号
text = text.translate(str.maketrans("", "", string.punctuation))
# 去除其他的标点符号
text = re.sub(r'[^\w\s]', '', text)
return text
# 测试
text = "Hello, world! This is a test."
text = remove_punctuation(text)
print(text)
```
输出结果为:`Hello world This is a test`
阅读全文
相关推荐
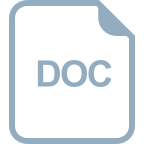
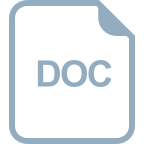
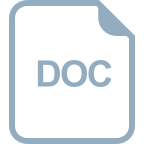
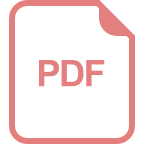












