c++线程中容器的使用
时间: 2024-01-08 18:03:15 浏览: 28
在C++中,如果多个线程需要同时访问容器,我们需要考虑容器的线程安全性,否则可能会导致数据竞争和异常。C++标准库提供了一些线程安全的容器,如`std::mutex`、`std::lock_guard`、`std::shared_mutex`、`std::atomic`等,可以用来保护容器。
下面以`std::vector`为例,介绍如何在多线程中使用容器:
```c++
#include <iostream>
#include <vector>
#include <thread>
#include <mutex>
std::mutex mtx; // 定义互斥锁
void push_vector(std::vector<int>& v, int n)
{
std::lock_guard<std::mutex> lck(mtx); // 定义互斥锁的lock_guard
for(int i=0; i<n; ++i)
v.push_back(i);
}
int main()
{
std::vector<int> v;
std::thread t1(push_vector, std::ref(v), 1000); // 启动线程1,往v中添加1000个元素
std::thread t2(push_vector, std::ref(v), 2000); // 启动线程2,往v中添加2000个元素
t1.join();
t2.join();
std::cout << "v size: " << v.size() << std::endl; // 输出v的元素个数
return 0;
}
```
在上面的代码中,我们定义了一个互斥锁`mtx`,并在`push_vector`函数中使用了`std::lock_guard`将互斥锁锁定,以保证每个线程对容器的访问是互斥的。在`main`函数中启动两个线程,分别往`v`中添加1000和2000个元素,最后输出`v`的元素个数。
除了使用互斥锁,还可以使用读写锁`std::shared_mutex`和原子类型`std::atomic`来保护容器的访问。在多线程编程中,需要谨慎处理数据竞争和死锁等问题,保证程序的正确性和稳定性。
相关推荐
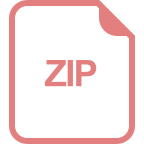
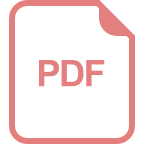














