C语言查询字符串中某字符串的个数
时间: 2023-04-10 10:02:27 浏览: 175
可以使用 strstr 函数来查找字符串中是否包含某个子字符串,然后使用循环来统计出现次数。以下是示例代码:
```c
#include <stdio.h>
#include <string.h>
int countSubstring(char *str, char *substr) {
int count = 0;
char *pos = str;
while ((pos = strstr(pos, substr)) != NULL) {
count++;
pos += strlen(substr);
}
return count;
}
int main() {
char str[] = "hello world, hello CSDN, hello C知道";
char substr[] = "hello";
int count = countSubstring(str, substr);
printf("'%s' 中包含 '%s' 的个数为 %d\n", str, substr, count);
return 0;
}
```
输出结果为:
```
'hello world, hello CSDN, hello C知道' 中包含 'hello' 的个数为 3
```
相关问题
C语言统计字符串中数字字符的个数
可以使用C语言中的循环和判断语句来统计字符串中数字字符的个数。具体方法如下:
```c
#include <stdio.h>
int main() {
char str[100];
int count = 0, i;
printf("请输入一个字符串:");
scanf("%s", str);
for (i = 0; str[i] != '\0'; i++) {
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
}
printf("字符串中数字字符的个数为:%d\n", count);
return 0;
}
```
这段代码首先定义了一个字符数组 `str` 和一个整型变量 `count`,用于存储输入的字符串和统计数字字符的个数。然后使用 `scanf()` 函数从标准输入中读取一个字符串。接下来使用 `for` 循环遍历字符串中的每个字符,如果字符是数字字符,则将计数器 `count` 加 1。最后输出统计结果。
希望可以帮到您!
C语言统计字符串中数字字符的个数。
可以用循环遍历字符串的每个字符,判断其是否是数字字符,如果是,则计数器加一。以下是代码示例:
```c
#include <stdio.h>
int main() {
char str[100];
int count = 0, i = 0;
printf("请输入一个字符串:");
fgets(str, 100, stdin); // 读入字符串
while (str[i] != '\0') {
if (str[i] >= '0' && str[i] <= '9') {
count++;
}
i++;
}
printf("字符串中数字字符的个数为:%d\n", count);
return 0;
}
```
以上代码中,循环遍历字符串中的每个字符,如果字符的 ASCII 码在数字字符的 ASCII 码范围内,则计数器加一。最后输出数字字符的个数。
相关推荐
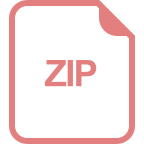
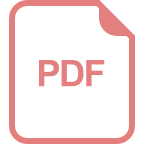
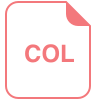
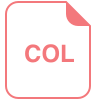
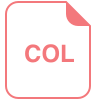
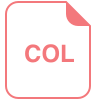
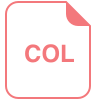







