给我一个c语言代码:建立单链表,并在单链表上实现插入、删除和查找操作
时间: 2023-05-31 15:07:29 浏览: 100
好的,以下是C语言代码,实现单链表的建立、插入、删除和查找操作:
#include <stdio.h>
#include <stdlib.h>
struct Node {
int data;
struct Node* next;
};
struct Node* create_linkedlist(int arr[], int size) {
struct Node* head = NULL;
struct Node* tail = NULL;
for (int i = 0; i < size; i++) {
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = arr[i];
new_node->next = NULL;
if (head == NULL) {
head = new_node;
tail = new_node;
} else {
tail->next = new_node;
tail = new_node;
}
}
return head;
}
void insert_node(struct Node* head, int index, int data) {
struct Node* new_node = (struct Node*) malloc(sizeof(struct Node));
new_node->data = data;
struct Node* curr_node = head;
for (int i = 0; i < index - 1; i++) {
curr_node = curr_node->next;
}
new_node->next = curr_node->next;
curr_node->next = new_node;
}
void delete_node(struct Node* head, int data) {
struct Node* curr_node = head;
struct Node* prev_node = NULL;
while (curr_node != NULL) {
if (curr_node->data == data) {
if (prev_node == NULL) {
head = curr_node->next;
} else {
prev_node->next = curr_node->next;
}
free(curr_node);
break;
}
prev_node = curr_node;
curr_node = curr_node->next;
}
}
struct Node* find_node(struct Node* head, int data) {
struct Node* curr_node = head;
while (curr_node != NULL) {
if (curr_node->data == data) {
return curr_node;
}
curr_node = curr_node->next;
}
return NULL;
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int size = sizeof(arr) / sizeof(arr[0]);
struct Node* head = create_linkedlist(arr, size);
insert_node(head, 2, 6);
delete_node(head, 4);
struct Node* found_node = find_node(head, 3);
if (found_node != NULL) {
printf("Found node with data %d\n", found_node->data);
} else {
printf("Node not found\n");
}
return 0;
}
阅读全文
相关推荐
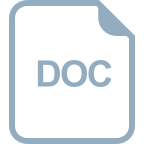
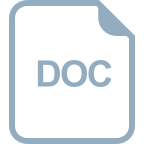
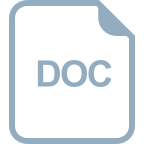




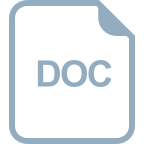
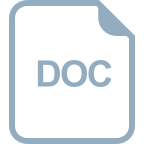
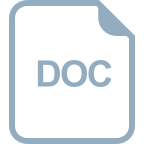
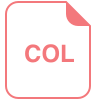







