springsecurity 实现验证码登录
时间: 2023-09-22 21:15:02 浏览: 171
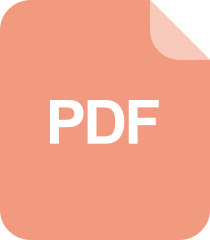
Spring Security 实现短信验证码登录功能
Spring Security可以通过自定义过滤器来实现验证码登录,主要步骤如下:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<dependency>
<groupId>com.google.code.kaptcha</groupId>
<artifactId>kaptcha</artifactId>
<version>2.3.2</version>
</dependency>
```
2. 配置验证码生成器
在Spring Security的配置类中定义验证码生成器:
```java
@Bean
public DefaultKaptcha kaptcha() {
Properties properties = new Properties();
properties.setProperty("kaptcha.border", "no");
properties.setProperty("kaptcha.textproducer.font.color", "black");
properties.setProperty("kaptcha.image.width", "150");
properties.setProperty("kaptcha.image.height", "50");
properties.setProperty("kaptcha.textproducer.char.length", "4");
Config config = new Config(properties);
DefaultKaptcha defaultKaptcha = new DefaultKaptcha();
defaultKaptcha.setConfig(config);
return defaultKaptcha;
}
```
3. 定义验证码过滤器
创建一个验证码过滤器类,继承`OncePerRequestFilter`,重写`doFilterInternal`方法,在其中判断是否需要验证验证码,如果是则验证并抛出异常。
```java
public class CaptchaFilter extends OncePerRequestFilter {
private final static String CAPTCHA_SESSION_KEY = "captcha_key";
private final static String LOGIN_URL = "/login";
@Autowired
private DefaultKaptcha kaptcha;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain)
throws ServletException, IOException {
if (LOGIN_URL.equals(request.getRequestURI()) && request.getMethod().equalsIgnoreCase("post")) {
try {
validate(request);
} catch (CaptchaException e) {
request.setAttribute("errorMessage", e.getMessage());
request.getRequestDispatcher("/login").forward(request, response);
return;
}
}
filterChain.doFilter(request, response);
}
private void validate(HttpServletRequest request) throws CaptchaException {
String captchaCode = request.getParameter("captchaCode");
String captchaSession = (String) request.getSession().getAttribute(CAPTCHA_SESSION_KEY);
if (StringUtils.isEmpty(captchaCode) || StringUtils.isEmpty(captchaSession)
|| !captchaCode.equalsIgnoreCase(captchaSession)) {
throw new CaptchaException("验证码错误");
}
}
}
```
其中`CaptchaException`为自定义异常类,用于抛出验证码校验失败的异常信息。
4. 注册验证码过滤器
将验证码过滤器注册到Spring Security的配置中:
```java
@Override
protected void configure(HttpSecurity http) throws Exception {
http.addFilterBefore(new CaptchaFilter(), UsernamePasswordAuthenticationFilter.class)
.authorizeRequests()
.antMatchers("/login", "/captcha").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/login").defaultSuccessUrl("/index")
.and()
.logout().logoutSuccessUrl("/login").permitAll()
.and()
.csrf().disable();
}
```
其中,`addFilterBefore`方法用于将验证码过滤器添加到Spring Security的过滤器链中。
5. 添加验证码生成接口
在控制器中添加一个生成验证码的接口,用于获取验证码图片和将验证码存储到session中:
```java
@GetMapping("/captcha")
public void captcha(HttpServletRequest request, HttpServletResponse response) throws IOException {
response.setHeader("Cache-Control", "no-store, no-cache");
response.setContentType("image/jpeg");
String captchaText = kaptcha.createText();
BufferedImage captchaImage = kaptcha.createImage(captchaText);
request.getSession().setAttribute(CAPTCHA_SESSION_KEY, captchaText);
ServletOutputStream outputStream = response.getOutputStream();
ImageIO.write(captchaImage, "jpg", outputStream);
IOUtils.closeQuietly(outputStream);
}
```
6. 修改登录页面
在登录页面中添加一个输入框和验证码图片:
```html
<form method="post" action="/login">
<div class="form-group">
<label for="username">用户名</label>
<input type="text" class="form-control" id="username" name="username" placeholder="请输入用户名">
</div>
<div class="form-group">
<label for="password">密码</label>
<input type="password" class="form-control" id="password" name="password" placeholder="请输入密码">
</div>
<div class="form-group">
<label for="captchaCode">验证码</label>
<div class="input-group">
<input type="text" class="form-control" id="captchaCode" name="captchaCode" placeholder="请输入验证码">
<div class="input-group-append">
<img src="/captcha" onclick="this.src='/captcha?'+Math.random()" style="cursor:pointer;">
</div>
</div>
</div>
<button type="submit" class="btn btn-primary">登录</button>
</form>
```
以上就是Spring Security实现验证码登录的步骤。
阅读全文
相关推荐
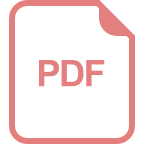
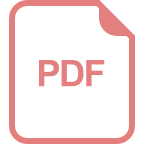
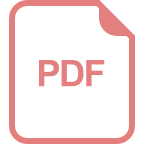
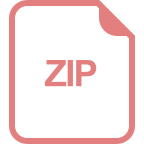
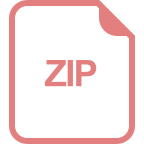
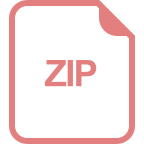
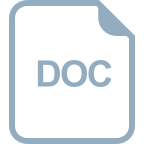
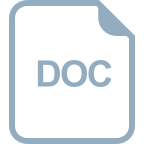









