解释 #include<iostream> using namespace std; int main() { int n=9; if(n++<10) cout<<n<<endl; else cout<<n--<<endl; return 0; }
时间: 2023-10-30 12:36:30 浏览: 202
这段代码的作用是输出数字 10。下面是代码的具体解释:
1. `#include<iostream>` 是 C++ 中用来包含输入输出流的头文件。
2. `using namespace std;` 是用来引入 std 命名空间的声明,这样就可以直接使用 cout 而不必写成 std::cout。
3. `int main()` 是程序的主函数。
4. `int n=9;` 定义了一个整数变量 n 并初始化为 9。
5. `if(n++<10) cout<<n<<endl;` 这一行代码中,先比较 n 和 10 的大小,由于 n 是 9,所以条件成立。然后 n 的值会被改变,变成 10,再输出 n 的值,所以输出结果为 10。
6. `else cout<<n--<<endl;` 这一行代码中,由于上一行代码已经执行了 n++,所以现在 n 的值是 10。但是由于条件成立,所以这一行代码不会执行,n 的值不会变化。
7. `return 0;` 表示程序运行结束,返回值为 0。
相关问题
改进代码#include<iostream> using namespace std; int main() { int n; cin >> n; cout << n << endl; return 0; }
您可以尝试使用更加规范的代码风格,例如:
```c++
#include <iostream>
using std::cin;
using std::cout;
using std::endl;
int main() {
int n;
cin >> n;
cout << n << endl;
return 0;
}
```
这样可以使代码更加易读,也符合 C++ 的风格规范。另外,如果您想要让程序更具健壮性,可以考虑增加对输入的检查,例如:
```c++
#include <iostream>
#include <limits>
using std::cin;
using std::cout;
using std::endl;
int main() {
int n;
while (!(cin >> n)) { // 如果输入不是一个整数,则重新输入
cin.clear(); // 清除错误标志
cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // 忽略缓冲区中的数据,直到遇到换行符
cout << "Invalid input, please try again." << endl;
}
cout << n << endl;
return 0;
}
```
这样可以避免用户输入非法数据导致程序崩溃的问题。
代码改错#include<iostream> using namespace std; int main() { int n; std::cin>>n; std::cout<<n<<endl; return 0; }
这段代码存在一个错误,就是在 return 语句后面的分号多了一个中文的分号符号,应该改成英文的分号符号。正确的代码如下:
```
#include<iostream>
using namespace std;
int main() {
int n;
cin >> n;
cout << n << endl;
return 0;
}
```
这样就能正确编译运行了。
阅读全文
相关推荐
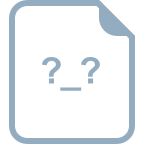














