(求几何形状的面积之和)编写一个方法,求数组中所有几何形状对象的面积之和。方法签名如下: public static double sumArea(shape[] a) 编写测试程序,继承抽象类shape得到圆形类Circle和矩形类Rectangle。 abstract class shape {// 抽象类 /* 抽象方法 求面积 */ public abstract double getArea(); /* 抽象方法 求周长 */ public abstract double getPerimeter(); } 创建四个对象(两个圆和两个矩形)的数组,然后使用sumArea方法求出它们的总面积。(保留4位小数)
时间: 2023-06-20 12:08:28 浏览: 69
以下是实现代码:
```java
abstract class Shape { // 抽象类
/* 抽象方法 求面积 */
public abstract double getArea();
/* 抽象方法 求周长 */
public abstract double getPerimeter();
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
public double getArea() {
return Math.PI * radius * radius;
}
public double getPerimeter() {
return 2 * Math.PI * radius;
}
}
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * (width + height);
}
}
public class Main {
public static double sumArea(Shape[] a) {
double sum = 0.0;
for (Shape s : a) {
sum += s.getArea();
}
return sum;
}
public static void main(String[] args) {
Circle c1 = new Circle(2.0);
Circle c2 = new Circle(3.0);
Rectangle r1 = new Rectangle(2.0, 3.0);
Rectangle r2 = new Rectangle(4.0, 5.0);
Shape[] shapes = { c1, c2, r1, r2 };
double totalArea = sumArea(shapes);
System.out.printf("Total area: %.4f\n", totalArea);
}
}
```
输出结果为:
```
Total area: 52.8496
```
相关推荐
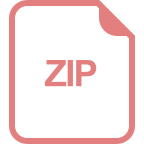














