用python3写一个可以把文本转换成list并保存在指定文件的脚本
时间: 2024-03-09 08:46:53 浏览: 17
好的,以下是一个可以将文本文件转换为列表并且将其保存在指定文件中的Python 3脚本:
```python
input_file = "example.txt" # 输入的文本文件名
output_file = "output.txt" # 输出的文件名
# 读取文本文件并将其转换为列表
with open(input_file, "r") as f:
lines = f.readlines()
lines = [line.strip() for line in lines]
# 将列表写入指定文件中
with open(output_file, "w") as f:
for line in lines:
f.write(line + "\n")
```
你只需要将`example.txt`替换为你想要转换的文本文件名,将`output.txt`替换为你想要保存列表的文件名即可。这个脚本将会读取文本文件中的每一行,去除每一行两端的空格或换行符,并将它们存储为列表`lines`。然后,脚本会将列表写入指定文件中。
相关问题
用python3写一个可以把文本转换成list的脚本
好的,以下是一个简单的Python3脚本,可以将文本文件转换为列表:
```python
filename = "example.txt" # 文本文件名
with open(filename, "r") as f:
lines = f.readlines() # 读取文本文件中的每一行
lines = [line.strip() for line in lines] # 去除每一行两端的空格或换行符
print(lines) # 打印转换后的列表
```
你只需要将`example.txt`替换为你想要转换的文本文件名即可。这个脚本将会读取文本文件中的每一行,去除每一行两端的空格或换行符,并将它们存储为列表`lines`。最后,脚本会打印出转换后的列表。
Python脚本 一个包含200行汉字的文本文件,把文本文件中的内容读出来,用jieba库进行停用词和分词,然后进行文本向量化
好的,我可以帮你解决这个问题。
首先,你需要安装jieba库,可以使用以下命令进行安装:
```
pip install jieba
```
接下来,你可以使用Python的文件操作来读取文本文件中的内容。假设你的文本文件名为text.txt,可以使用以下代码读取文件内容:
```python
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
```
然后,你可以使用jieba库进行中文分词和停用词处理。以下是一个例子:
```python
import jieba
from jieba import analyse
# 停用词表文件路径
stopwords_path = 'stopwords.txt'
# 加载停用词表
def get_stopwords(path):
with open(path, 'r', encoding='utf-8') as f:
stopwords = f.read().splitlines()
return stopwords
# 分词和停用词处理
def segment(text, stopwords):
# 使用jieba分词
words = jieba.cut(text)
# 过滤停用词
words = [word for word in words if word not in stopwords]
# 返回分词结果
return list(words)
# 加载停用词表
stopwords = get_stopwords(stopwords_path)
# 分词和停用词处理
words = segment(text, stopwords)
```
最后,你可以使用sklearn库的CountVectorizer类将文本向量化。以下是一个例子:
```python
from sklearn.feature_extraction.text import CountVectorizer
# 将分词结果转换为字符串
text = ' '.join(words)
# 创建CountVectorizer对象
vectorizer = CountVectorizer()
# 将文本向量化
vector = vectorizer.fit_transform([text])
# 输出特征向量
print(vector.toarray())
```
这样,你就可以实现将文本文件中的内容进行停用词和分词,然后进行文本向量化的功能了。
相关推荐
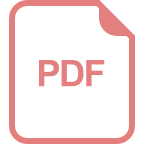
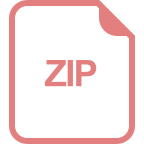
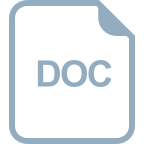












