编写一个 Python 脚本,读取 `example.txt` 文件中的内容,并统计文件中每个单词出现的次数。最后将结果以字典的形式打印出来。
时间: 2024-11-25 20:10:16 浏览: 4
当然,我们可以使用Python的内置模块`collections`中的`Counter`来实现这个功能。下面是一个简单的脚本示例:
```python
from collections import Counter
# 读取example.txt文件的内容
with open('example.txt', 'r') as file:
text = file.read().lower()
# 使用split()方法按空格分割字符串成单词列表
words_list = text.split()
# 使用Counter计算每个单词的频率
word_counts = Counter(words_list)
# 打印结果
print("单词及其出现次数:")
for word, count in word_counts.items():
print(f"{word}: {count}")
```
在这个脚本中,我们首先打开`example.txt`文件,读取所有文本,并将其转换为小写以忽略大小写差异。然后,我们将文本分割成单词列表,再使用`Counter`来统计每个单词的出现次数。最后,遍历并打印出每个单词及其相应的频率。
运行这个脚本后,你会看到`example.txt`文件中每个单词及其出现次数的结果。
相关问题
编写一个python脚本,读取‘example.txt’文件中的内容,并统计文件中每个单词出现的次数,最后将结果以字典形式打印出来
当然可以。在Python中,我们可以使用内置模块`collections`中的`Counter`类来轻松地统计文本文件中每个单词的出现次数。下面是一个简单的脚本示例:
```python
import string
from collections import Counter
# 定义函数来处理文件内容
def count_words_in_file(file_name):
# 打开文件并读取所有行
with open(file_name, 'r', encoding='utf-8') as file:
content = file.read()
# 移除标点符号并将内容转换为小写
words = content.translate(str.maketrans('', '', string.punctuation)).lower().split()
# 使用Counter计算每个单词的频率
word_count = Counter(words)
# 打印结果字典
for word, frequency in word_count.items():
print(f"{word}: {frequency}")
# 调用函数处理'example.txt'文件
count_words_in_file('example.txt')
```
这个脚本会打开指定的文件,去除其中的标点符号并转为小写,然后使用`Counter`统计单词出现的次数,最后输出每个单词及其对应的频率。
编写一个Python 脚本,读取example.txt文件中的每一行,并将每一行的内容前面加上行号,然后将处理后的内容写入到一个新文件、numbered_example.txt’中。并且打印例如: 1: Hello,World!2: This is a test file.
你可以使用Python的内置模块`open()`来读取文件,`enumerate()`函数来生成行号,以及`with open()`来处理文件的读写。下面是一个简单的脚本示例:
```python
# 打开源文件和目标文件
with open('example.txt', 'r') as f_in, open('numbered_example.txt', 'w') as f_out:
# 遍历源文件中的每一行
for i, line in enumerate(f_in, start=1): # start参数从1开始计数
# 在每行前添加行号
processed_line = f"{i}: {line.strip()}"
# 写入处理后的行到目标文件
f_out.write(processed_line + '\n')
# 打印例子
print("1:", "Hello,World!")
print("2:", "This is a test file.")
```
这个脚本会先读取`example.txt`文件的每一行,然后在行前加上对应的行号并写入新的`numbered_example.txt`文件中。最后,我们手动演示了如何在脚本外部打印这两个例子。
阅读全文
相关推荐
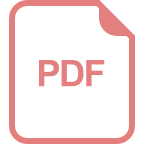
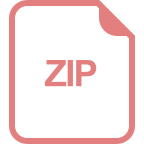
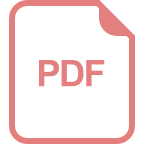














