利用反射实现DataTable 与 List<T> 转换
时间: 2024-03-01 14:49:36 浏览: 18
好的,我可以回答你的问题。利用反射可以实现 DataTable 与 List<T> 之间的转换。
首先,我们需要定义一个通用的方法,该方法可以将 List<T> 转换为 DataTable,也可以将 DataTable 转换为 List<T>。该方法的实现需要借助反射机制。
下面是将 List<T> 转换为 DataTable 的代码:
```csharp
public static DataTable ConvertToDataTable<T>(List<T> list)
{
DataTable table = new DataTable();
PropertyInfo[] properties = typeof(T).GetProperties();
foreach (PropertyInfo property in properties)
{
table.Columns.Add(property.Name, property.PropertyType);
}
foreach (T item in list)
{
DataRow row = table.NewRow();
foreach (PropertyInfo property in properties)
{
row[property.Name] = property.GetValue(item, null);
}
table.Rows.Add(row);
}
return table;
}
```
上面的代码中,我们首先使用反射机制获取类型 T 的所有属性信息,然后使用这些属性信息创建 DataTable 的列。接着,我们遍历 List<T> 中的每个元素,使用反射获取元素的属性值,并将这些属性值添加到 DataRow 中,最后将 DataRow 添加到 DataTable 中。
下面是将 DataTable 转换为 List<T> 的代码:
```csharp
public static List<T> ConvertToList<T>(DataTable table) where T : new()
{
List<T> list = new List<T>();
PropertyInfo[] properties = typeof(T).GetProperties();
foreach (DataRow row in table.Rows)
{
T item = new T();
foreach (PropertyInfo property in properties)
{
if (table.Columns.Contains(property.Name))
{
property.SetValue(item, row[property.Name], null);
}
}
list.Add(item);
}
return list;
}
```
上面的代码中,我们同样使用反射机制获取类型 T 的所有属性信息,然后遍历 DataTable 中的每个 DataRow,使用反射设置每个 DataRow 中对应的属性值,并将 T 对象添加到 List<T> 中。
希望这些代码可以帮助你解决问题。
相关推荐
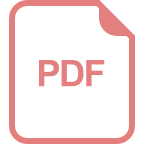









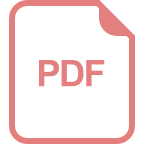
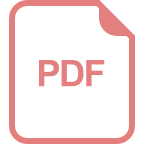
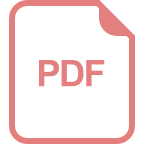
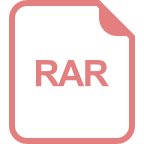
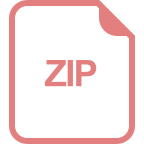