python实现图片rgb通道分离并将分离的rgb通道绘制成饼图
时间: 2024-05-12 22:13:06 浏览: 82
可以使用Python的Pillow库来实现图片rgb通道分离,并使用matplotlib库来绘制饼图。
以下是示例代码:
```python
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
# 打开图片
img = Image.open('test.jpg')
# 将图片转换成numpy数组
img_array = np.array(img)
# 分离RGB通道
red_channel = img_array[:, :, 0]
green_channel = img_array[:, :, 1]
blue_channel = img_array[:, :, 2]
# 统计像素值
red_count = np.count_nonzero(red_channel)
green_count = np.count_nonzero(green_channel)
blue_count = np.count_nonzero(blue_channel)
# 计算比例
total_count = red_count + green_count + blue_count
red_percent = red_count / total_count * 100
green_percent = green_count / total_count * 100
blue_percent = blue_count / total_count * 100
# 绘制饼图
labels = ['Red', 'Green', 'Blue']
sizes = [red_percent, green_percent, blue_percent]
colors = ['red', 'green', 'blue']
plt.pie(sizes, labels=labels, colors=colors, autopct='%1.1f%%', startangle=90)
plt.axis('equal')
plt.title('RGB Channels')
plt.show()
```
注意:在运行代码之前,需要先安装Pillow和matplotlib库。可以使用以下命令来安装:
```
pip install Pillow matplotlib
```
同时,代码中的`test.jpg`需要替换成实际的图片路径。
阅读全文
相关推荐
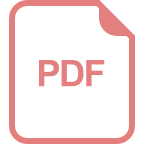
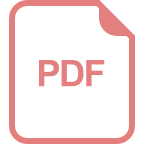
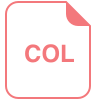
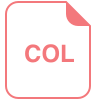
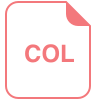
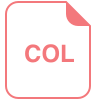
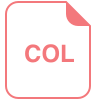
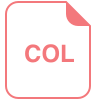
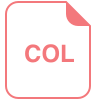
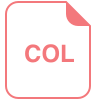
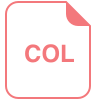
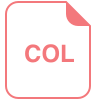



