基于Springboot框架写一个接口,需求是使用WebClient异步的方式向其他接口发送http请求,并一共监听十分钟的响应结果,然后将相应结果保存到数据库
时间: 2024-05-09 13:18:44 浏览: 9
首先,需要添加WebClient和数据库相关的依赖:
```xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-webflux</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-mongodb-reactive</artifactId>
</dependency>
</dependencies>
```
接下来,创建一个RestController:
```java
@RestController
public class AsyncController {
private final WebClient webClient;
private final ReactiveMongoTemplate mongoTemplate;
public AsyncController(WebClient.Builder webClientBuilder, ReactiveMongoTemplate mongoTemplate) {
this.webClient = webClientBuilder.build();
this.mongoTemplate = mongoTemplate;
}
@GetMapping("/async")
public Mono<String> async() {
LocalDateTime startTime = LocalDateTime.now();
System.out.println("Start time: " + startTime);
Mono<String> result = webClient.get()
.uri("http://localhost:8080/some-api")
.retrieve()
.bodyToMono(String.class)
.timeout(Duration.ofMinutes(10))
.doOnSuccess(response -> {
LocalDateTime endTime = LocalDateTime.now();
System.out.println("End time: " + endTime);
System.out.println("Response: " + response);
MongoDoc doc = new MongoDoc(startTime, endTime, response);
mongoTemplate.insert(doc).subscribe();
});
return result;
}
private static class MongoDoc {
private LocalDateTime startTime;
private LocalDateTime endTime;
private String response;
public MongoDoc(LocalDateTime startTime, LocalDateTime endTime, String response) {
this.startTime = startTime;
this.endTime = endTime;
this.response = response;
}
// getters and setters
}
}
```
在构造函数中注入WebClient和ReactiveMongoTemplate,然后在async()方法中使用WebClient发送请求,并在响应结果中处理保存到数据库的逻辑。
MongoDoc是一个简单的POJO对象,用于保存响应结果的信息,其可以通过ReactiveMongoTemplate插入到MongoDB中。
需要注意的是,由于使用了WebClient异步发送请求,所以async()方法的返回值类型需要是Mono<String>,而不是普通的String类型。同时,也需要使用timeout()方法指定请求的超时时间为10分钟。
最后,可以通过发送GET请求到http://localhost:8080/async来测试该接口。如果一切正常,那么将会在十分钟后收到响应,并将响应结果保存到MongoDB中。
相关推荐
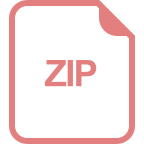
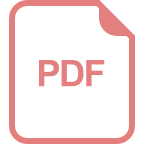














