用抖音开发者工具开发坦克大战小游戏,显示完整代码
时间: 2023-06-24 10:08:43 浏览: 112
抖音开发者工具可以用来开发小程序,无法直接开发小游戏。不过可以使用类似的开发工具,例如微信小游戏开发者工具或者百度小游戏开发者工具。以下是一个简单的坦克大战小游戏的示例代码:
```javascript
// 游戏画布宽度
const WIDTH = 320;
// 游戏画布高度
const HEIGHT = 480;
// 创建游戏画布
const canvas = wx.createCanvas();
canvas.width = WIDTH;
canvas.height = HEIGHT;
// 获取画布上下文
const ctx = canvas.getContext('2d');
// 坦克对象
const tank = {
x: 0,
y: 0,
direction: 'up',
speed: 2,
draw() {
// 根据方向画出坦克
switch (this.direction) {
case 'up':
ctx.fillRect(this.x, this.y, 20, 30);
ctx.fillRect(this.x + 5, this.y + 30, 10, 10);
break;
case 'down':
ctx.fillRect(this.x, this.y + 10, 20, 30);
ctx.fillRect(this.x + 5, this.y, 10, 10);
break;
case 'left':
ctx.fillRect(this.x, this.y, 30, 20);
ctx.fillRect(this.x + 30, this.y + 5, 10, 10);
break;
case 'right':
ctx.fillRect(this.x + 10, this.y, 30, 20);
ctx.fillRect(this.x, this.y + 5, 10, 10);
break;
}
},
move() {
// 根据方向移动坦克
switch (this.direction) {
case 'up':
this.y -= this.speed;
break;
case 'down':
this.y += this.speed;
break;
case 'left':
this.x -= this.speed;
break;
case 'right':
this.x += this.speed;
break;
}
}
};
// 监听键盘事件
wx.onKeyDown(function(event) {
switch (event.keyCode) {
case 37:
// 左箭头键
tank.direction = 'left';
break;
case 38:
// 上箭头键
tank.direction = 'up';
break;
case 39:
// 右箭头键
tank.direction = 'right';
break;
case 40:
// 下箭头键
tank.direction = 'down';
break;
}
});
// 游戏循环
function gameLoop() {
// 清空画布
ctx.clearRect(0, 0, WIDTH, HEIGHT);
// 移动坦克
tank.move();
// 画出坦克
tank.draw();
// 继续下一帧
requestAnimationFrame(gameLoop);
}
// 开始游戏循环
gameLoop();
```
以上代码是一个非常简单的示例,只有一个坦克可以移动,没有敌人和子弹等元素。如果要实现完整的坦克大战游戏,需要更多的代码和逻辑。
相关推荐
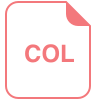
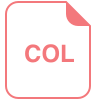
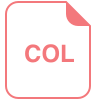
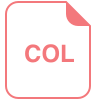
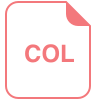









