这段代码为什么发生死锁:pthread_mutex_t mutexA = PTHREAD_MUTEX_INITIALIZER; pthread_mutex_t mutexB = PTHREAD_MUTEX_INITIALIZER; pthread_mutex_t mutexC = PTHREAD_MUTEX_INITIALIZER; static int counterA = 0; static int counterB = 0; int func1() { pthread_mutex_lock(&mutexA); ++counterA; sleep(1); pthread_mutex_lock(&mutexB); ++counterB; pthread_mutex_unlock(&mutexB); pthread_mutex_unlock(&mutexA); return counterA; } int func2() { pthread_mutex_lock(&mutexB); ++counterB; sleep(1); pthread_mutex_lock(&mutexA); ++counterA; pthread_mutex_unlock(&mutexA); pthread_mutex_unlock(&mutexB); return counterB; } void* start_routine1(void* arg) { while (1) { int iRetValue = func1(); if (iRetValue == 100000) { pthread_exit(NULL); } } } void* start_routine2(void* arg) { while (1) { int iRetValue = func2(); if (iRetValue == 100000) { pthread_exit(NULL); } } } void* start_routine(void* arg) { while (1) { sleep(1); char szBuf[128]; memset(szBuf, 0, sizeof(szBuf)); strcpy(szBuf, (char*)arg); } } int main() { pthread_t tid[4]; if (pthread_create(&tid[0], NULL, &start_routine1, NULL) != 0) { _exit(1); } if (pthread_create(&tid[1], NULL, &start_routine2, NULL) != 0) { _exit(1); } if (pthread_create(&tid[2], NULL, &start_routine, "thread3") != 0) { _exit(1); } if (pthread_create(&tid[3], NULL, &start_routine, "thread3") != 0) { _exit(1); } sleep(5); //pthread_cancel(tid[0]); pthread_join(tid[0], NULL); pthread_join(tid[1], NULL); pthread_join(tid[2], NULL); pthread_join(tid[3], NULL); pthread_mutex_destroy(&mutexA); pthread_mutex_destroy(&mutexB); pthread_mutex_destroy(&mutexC); return 0; }
时间: 2023-06-12 11:05:57 浏览: 154
这段代码可能会发生死锁的原因是,当两个线程同时尝试获得多个互斥锁时,可能会发生死锁。例如,如果一个线程在获得 mutexA 的锁之后,尝试获得 mutexB 的锁,而另一个线程已经获得了 mutexB 的锁并正在尝试获得 mutexA 的锁,那么两个线程将会相互等待对方释放已经获取的锁,导致死锁。
解决死锁的方法是,保证所有线程获取多个互斥锁的顺序是相同的。例如,可以规定所有线程都先获取 mutexA 的锁,再获取 mutexB 的锁,最后获取 mutexC 的锁。这样做可以避免死锁的发生。
相关问题
pthread_mutex_trylock用法
`pthread_mutex_trylock` 函数用于尝试对一个互斥锁进行加锁,如果该锁当前没有被其他线程占用,则加锁成功,返回0;否则,加锁失败,返回EBUSY。其函数原型如下:
```c
#include <pthread.h>
int pthread_mutex_trylock(pthread_mutex_t *mutex);
```
其中,`mutex` 参数是指向互斥锁变量的指针。
使用 `pthread_mutex_trylock` 时需要注意以下几点:
1. 确保互斥锁已经被初始化,否则加锁行为是未定义的。
2. 如果一个线程已经拥有了该互斥锁,再次尝试对该锁进行加锁会导致死锁。
3. 在加锁成功后,必须在合适的时候调用 `pthread_mutex_unlock` 函数对该锁进行解锁,否则会导致其他线程永远无法对该锁进行加锁。
下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
void* thread_func(void* arg) {
int ret = pthread_mutex_trylock(&mutex);
if (ret == 0) {
printf("Thread %ld got the lock.\n", (long)arg);
pthread_mutex_unlock(&mutex);
} else {
printf("Thread %ld failed to get the lock.\n", (long)arg);
}
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_create(&thread1, NULL, thread_func, (void*)1);
pthread_create(&thread2, NULL, thread_func, (void*)2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
return 0;
}
```
该程序创建了两个线程分别尝试对互斥锁进行加锁。其中,一个线程能够成功获取到锁,另一个线程则失败。运行结果类似如下:
```
Thread 1 got the lock.
Thread 2 failed to get the lock.
```
if(threadIsExist) { int existCode; waitpid(ret,&existCode,0); if(kill(ret,existCode)) { ret = pthread_create(&tid,NULL,&SendRtpProc,(LPVOID)&media_para); } else { printf("terminateTif(ret > 0) { threadIsExist = TRUE; }优化这段代码
在优化这段代码之前,需要先了解该代码的具体功能和运行环境,以便更好地进行优化。可能需要回答以下问题:
- 这段代码在什么情况下会被执行?
- `threadIsExist`变量的作用是什么?
- `SendRtpProc`函数的具体功能是什么?
- 是否存在多线程间的数据竞争问题?
假设已经回答了上述问题,并且确认该代码在多线程环境下运行,存在数据竞争问题。那么可以考虑使用互斥锁来解决数据竞争问题。
下面是一种可能的优化方案:
```c
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER; // 定义一个静态互斥锁
if (threadIsExist) {
pthread_mutex_lock(&mutex); // 加锁
// 等待线程退出
int existCode;
waitpid(ret, &existCode, 0);
// 根据退出码判断线程是否正常退出,如果没有则重新创建一个线程
if (kill(ret, existCode)) {
ret = pthread_create(&tid, NULL, &SendRtpProc, (LPVOID)&media_para);
} else {
printf("Thread exited normally.\n");
}
threadIsExist = true;
pthread_mutex_unlock(&mutex); // 解锁
}
```
在这个优化方案中,首先定义了一个静态互斥锁`mutex`,用来保护共享变量`threadIsExist`的读写操作。当需要读写`threadIsExist`变量时,需要先调用`pthread_mutex_lock`函数来加锁,以避免其他线程同时访问该变量。在修改完变量后,需要调用`pthread_mutex_unlock`函数来解锁。
在具体实现中,先调用`waitpid`函数等待线程退出,并获取退出码`existCode`。然后,根据退出码判断线程是否正常退出。如果没有正常退出,则调用`kill`函数杀死线程,并重新创建一个新的线程。如果线程已经正常退出,则不需要重新创建一个新的线程。最后,将`threadIsExist`变量设置为true,表示已经有一个线程在运行。
需要注意的是,以上代码仅仅是一种优化方案,具体实现可能需要根据实际情况进行调整和修改。例如,如果`SendRtpProc`函数中存在需要释放的资源,需要在线程退出前进行相应的清理。同时,在使用互斥锁时,需要考虑锁的粒度和性能问题,以避免出现死锁或性能瓶颈。
阅读全文
相关推荐
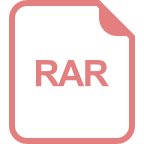
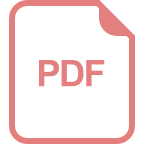
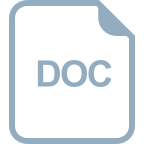
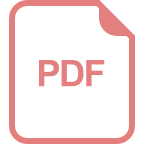
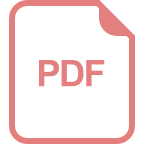
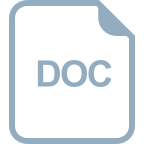
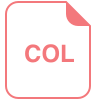
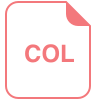
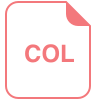
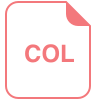
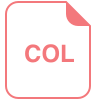
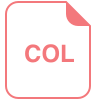
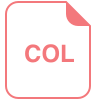


