请定义一个计算长方形面积rectangle函数,包含两个实型参数lenwidth,len表示长,width表示宽,将计算结果返回。
时间: 2023-10-02 22:06:04 浏览: 100
下面是一个计算长方形面积的rectangle函数的定义:
```python
def rectangle(len, width):
area = len * width
return area
```
其中,`len`和`width`是函数的两个参数,分别表示长和宽。在函数内部,我们使用这两个参数计算长方形的面积,并将结果保存在变量`area`中。最后,我们使用`return`语句将计算结果返回。
相关问题
用请定义一个计算长方形面积rectangle函数,包含两个实型参数lenwidth,len表示长,width表示宽,将计算结果返回。
下面是一个使用 Python 语言定义计算长方形面积的函数:
```python
def rectangle(len, width):
area = len * width
return area
```
其中,函数名为 `rectangle`,接收两个参数 `len` 和 `width`,并将它们相乘得到长方形的面积 `area`,最后将面积作为返回值返回。
用C++定义一个Rectangle类,长方形类有四个点对象作为属性,编写方法实现以下功能: 判断四个点能不能成为一个长方形的四个顶点,计算出长方形的长,计算出长方形的宽,计算长方形的面积,计算长方形的周长
好的,下面是C++定义的Rectangle类的代码:
```cpp
#include <iostream>
#include <cmath>
using namespace std;
class Point {
public:
int x;
int y;
};
class Rectangle {
public:
Point p1, p2, p3, p4;
Rectangle(Point _p1, Point _p2, Point _p3, Point _p4);
bool isRectangle();
double getLength();
double getWidth();
double getArea();
double getPerimeter();
};
Rectangle::Rectangle(Point _p1, Point _p2, Point _p3, Point _p4) {
p1 = _p1;
p2 = _p2;
p3 = _p3;
p4 = _p4;
}
bool Rectangle::isRectangle() {
// 判断四个点能否组成长方形
double len1 = sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
double len2 = sqrt(pow(p1.x - p3.x, 2) + pow(p1.y - p3.y, 2));
double len3 = sqrt(pow(p1.x - p4.x, 2) + pow(p1.y - p4.y, 2));
if (len1 == len2 && len3 == len2 && len1 != 0 && len3 != 0) {
return true;
}
return false;
}
double Rectangle::getLength() {
// 计算长方形的长
double len1 = sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
double len2 = sqrt(pow(p1.x - p3.x, 2) + pow(p1.y - p3.y, 2));
double len3 = sqrt(pow(p1.x - p4.x, 2) + pow(p1.y - p4.y, 2));
if (len1 == len2 && len3 == len2 && len1 != 0 && len3 != 0) {
return len1;
}
return 0;
}
double Rectangle::getWidth() {
// 计算长方形的宽
double len1 = sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
double len2 = sqrt(pow(p1.x - p3.x, 2) + pow(p1.y - p3.y, 2));
double len3 = sqrt(pow(p1.x - p4.x, 2) + pow(p1.y - p4.y, 2));
if (len1 == len2 && len3 == len2 && len1 != 0 && len3 != 0) {
double width1 = sqrt(pow(p2.x - p3.x, 2) + pow(p2.y - p3.y, 2));
double width2 = sqrt(pow(p2.x - p4.x, 2) + pow(p2.y - p4.y, 2));
if (width1 == width2 && width1 != 0 && width2 != 0) {
return width1;
}
}
return 0;
}
double Rectangle::getArea() {
// 计算长方形的面积
double length = getLength();
double width = getWidth();
if (length != 0 && width != 0) {
return length * width;
}
return 0;
}
double Rectangle::getPerimeter() {
// 计算长方形的周长
double length = getLength();
double width = getWidth();
if (length != 0 && width != 0) {
return 2 * (length + width);
}
return 0;
}
int main() {
Point p1 = {0, 0};
Point p2 = {0, 1};
Point p3 = {2, 0};
Point p4 = {2, 1};
Rectangle r(p1, p2, p3, p4);
if (r.isRectangle()) {
cout << "该四个点能构成长方形" << endl;
cout << "长:" << r.getLength() << endl;
cout << "宽:" << r.getWidth() << endl;
cout << "面积:" << r.getArea() << endl;
cout << "周长:" << r.getPerimeter() << endl;
} else {
cout << "该四个点不能构成长方形" << endl;
}
return 0;
}
```
在上面的代码中,我们定义了两个类:Point(点)和Rectangle(长方形)。Point类表示一个二维平面上的点,包括x和y两个坐标属性。Rectangle类表示一个长方形,包括四个点对象作为属性。
在Rectangle类中,我们实现了以下方法:
- 构造函数:初始化四个点对象;
- isRectangle()方法:判断四个点能否组成长方形;
- getLength()方法:计算长方形的长;
- getWidth()方法:计算长方形的宽;
- getArea()方法:计算长方形的面积;
- getPerimeter()方法:计算长方形的周长。
在主函数中,我们定义了四个点,并使用这四个点初始化Rectangle对象。如果这四个点能组成长方形,则输出长、宽、面积和周长。否则,输出“该四个点不能构成长方形”。
阅读全文
相关推荐
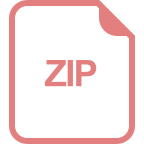
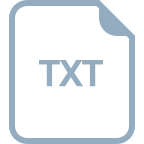









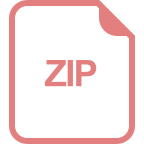