C语言小子集的词法分析程序
时间: 2024-05-07 08:19:56 浏览: 82
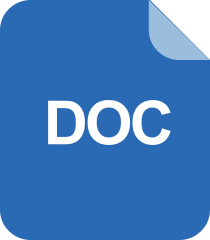
编译原理 词法分析程序

以下是一个简单的C语言小子集的词法分析程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
#define MAX_TOKEN_LENGTH 100
enum TokenType {
KEYWORD,
IDENTIFIER,
NUMBER,
OPERATOR,
PUNCTUATION
};
struct Token {
enum TokenType type;
char value[MAX_TOKEN_LENGTH];
};
int is_keyword(char* token) {
char* keywords[] = {"int", "float", "char", "if", "else", "while", "for", "return", "void"};
int num_keywords = sizeof(keywords) / sizeof(keywords[0]);
for (int i = 0; i < num_keywords; i++) {
if (strcmp(token, keywords[i]) == 0) {
return 1;
}
}
return 0;
}
int is_operator(char c) {
char* operators = "+-*/%=";
for (int i = 0; i < strlen(operators); i++) {
if (c == operators[i]) {
return 1;
}
}
return 0;
}
int is_punctuation(char c) {
char* punctuations = "(){}[];,";
for (int i = 0; i < strlen(punctuations); i++) {
if (c == punctuations[i]) {
return 1;
}
}
return 0;
}
struct Token get_token(char* input, int* pos) {
struct Token token;
token.type = -1;
token.value[0] = '\0';
int len = strlen(input);
while (*pos < len && isspace(input[*pos])) {
(*pos)++;
}
if (*pos == len) {
return token;
}
if (isalpha(input[*pos])) {
token.type = IDENTIFIER;
int i = 0;
while (*pos + i < len && isalnum(input[*pos + i])) {
token.value[i] = input[*pos + i];
i++;
if (i == MAX_TOKEN_LENGTH) {
break;
}
}
token.value[i] = '\0';
if (is_keyword(token.value)) {
token.type = KEYWORD;
}
} else if (isdigit(input[*pos])) {
token.type = NUMBER;
int i = 0;
while (*pos + i < len && isdigit(input[*pos + i])) {
token.value[i] = input[*pos + i];
i++;
if (i == MAX_TOKEN_LENGTH) {
break;
}
}
token.value[i] = '\0';
} else if (is_operator(input[*pos])) {
token.type = OPERATOR;
token.value[0] = input[*pos];
token.value[1] = '\0';
(*pos)++;
} else if (is_punctuation(input[*pos])) {
token.type = PUNCTUATION;
token.value[0] = input[*pos];
token.value[1] = '\0';
(*pos)++;
} else {
// unrecognized character
(*pos)++;
}
return token;
}
int main() {
char input[] = "int main() {\n return 0;\n}";
int pos = 0;
struct Token token;
do {
token = get_token(input, &pos);
if (token.type != -1) {
printf("Type: %d, Value: %s\n", token.type, token.value);
}
} while (token.type != -1);
return 0;
}
```
该程序实现了简单的C语言小子集的词法分析功能,可以分别识别出关键字、标识符、数字、运算符和标点符号,并输出它们的类型和值。在实现中,使用了一个结构体 Token 来表示识别出的 token,其中包括类型和值两个属性。识别过程通过 get_token 函数实现,该函数接受输入字符串和当前位置作为参数,返回识别出的 token。在主函数中,不断调用 get_token 函数直到识别出一个未知类型的 token,输出识别结果。
阅读全文
相关推荐
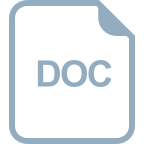
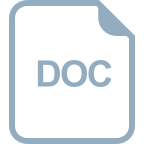
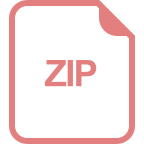

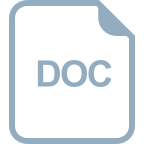
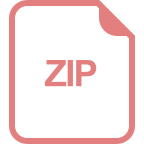
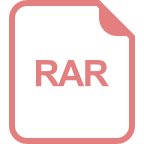
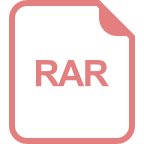
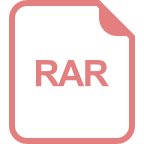
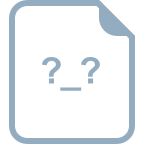
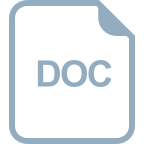
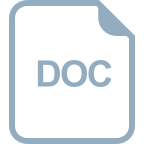
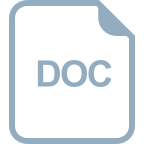
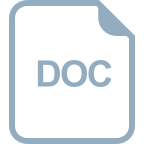
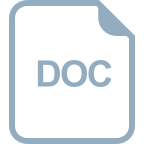

