用Python写出完成如下功能的代码:生成包含10000个样本数据集,每个样本包含10个特征;对数据进行预处理;特征之间相关性分析;特征选择;构建决策树分类模型;优化超参数;预测;测试模型的性能;
时间: 2024-05-08 20:15:14 浏览: 104
生成数据集:
```python
import numpy as np
# 生成10000个样本,每个样本包含10个特征
X = np.random.rand(10000, 10)
# 生成10000个标签,0或1
y = np.random.randint(0, 2, size=10000)
```
预处理数据:
```python
from sklearn.preprocessing import StandardScaler
# 标准化特征
scaler = StandardScaler()
X = scaler.fit_transform(X)
```
特征之间相关性分析:
```python
import seaborn as sns
import matplotlib.pyplot as plt
# 计算相关系数矩阵
corr = np.corrcoef(X.T)
# 绘制相关系数热力图
plt.figure(figsize=(10, 10))
sns.heatmap(corr, annot=True, cmap='coolwarm')
```
特征选择:
```python
from sklearn.feature_selection import SelectKBest, f_classif
# 选择最好的5个特征
selector = SelectKBest(score_func=f_classif, k=5)
X_new = selector.fit_transform(X, y)
```
构建决策树分类模型:
```python
from sklearn.tree import DecisionTreeClassifier
# 构建决策树分类模型
clf = DecisionTreeClassifier(random_state=42)
clf.fit(X_new, y)
```
优化超参数:
```python
from sklearn.model_selection import GridSearchCV
# 定义超参数搜索范围
param_grid = {
'max_depth': [2, 4, 6, 8],
'min_samples_split': [2, 4, 6, 8],
'min_samples_leaf': [1, 2, 3, 4],
'criterion': ['gini', 'entropy']
}
# 定义网格搜索对象
grid_search = GridSearchCV(clf, param_grid=param_grid, cv=5, n_jobs=-1)
# 进行网格搜索
grid_search.fit(X_new, y)
# 打印最佳超参数组合
print(grid_search.best_params_)
```
预测:
```python
# 预测测试集
y_pred = grid_search.predict(X_new_test)
```
测试模型性能:
```python
from sklearn.metrics import accuracy_score, classification_report
# 计算准确率
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy: {:.2f}%".format(accuracy * 100))
# 打印分类报告
print(classification_report(y_test, y_pred))
```
阅读全文
相关推荐
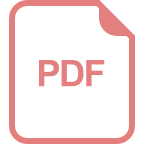
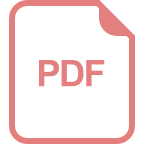
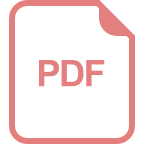
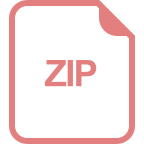
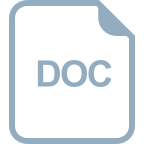


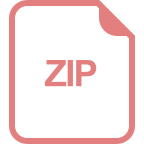
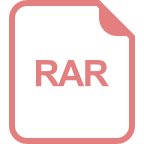
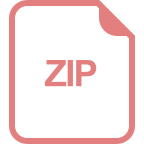
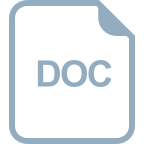
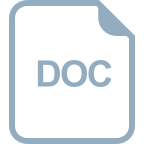
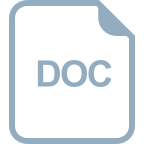


