openlayer加载视频
时间: 2023-04-09 22:00:49 浏览: 99
我可以回答这个问题。OpenLayers可以通过使用Video源来加载视频。您可以使用Video源来加载本地或在线视频。您可以使用以下代码来加载视频:
var video = new ol.source.Video({
url: 'path/to/video.mp4',
attributions: [
new ol.Attribution({
html: 'Video provided by <a href="https://www.example.com/">Example.com</a>'
})
]
});
var videoLayer = new ol.layer.Image({
source: video
});
map.addLayer(videoLayer);
请注意,您需要使用适当的视频格式和编解码器来确保视频能够正确加载。
相关问题
yolov8读取视频
很抱歉,yolov8并不是一个已知的模型或库,可能是您自己开发的模型或者笔误。如果您是想问如何使用YOLOv3或YOLOv4读取视频,可以使用OpenCV库中的VideoCapture类来实现。以下是一个使用YOLOv4读取视频并进行目标检测的示例代码:
```python
import cv2
import numpy as np
# 加载YOLOv4模型
net = cv2.dnn.readNetFromDarknet('yolov4.cfg', 'yolov4.weights')
net.setPreferableBackend(cv2.dnn.DNN_BACKEND_CUDA)
net.setPreferableTarget(cv2.dnn.DNN_TARGET_CUDA)
# 加载类别标签
classes = []
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 读取视频
cap = cv2.VideoCapture('test.mp4')
while True:
# 读取一帧
ret, frame = cap.read()
if not ret:
break
# 对帧进行目标检测
blob = cv2.dnn.blobFromImage(frame, 1/255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * frame.shape[1])
center_y = int(detection[1] * frame.shape[0])
w = int(detection[2] * frame.shape[1])
h = int(detection[3] * frame.shape[0])
x = int(center_x - w/2)
y = int(center_y - h/2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 绘制检测结果
for i in indices:
i = i[0]
x, y, w, h = boxes[i]
label = classes[class_ids[i]]
confidence = confidences[i]
color = (0, 255, 0)
cv2.rectangle(frame, (x, y), (x+w, y+h), color, 2)
cv2.putText(frame, f'{label} {confidence:.2f}', (x, y-10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 显示结果
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
yolo逐帧检测后输出视频
要实现yolo逐帧检测后输出视频,您可以按照以下步骤进行操作:
1. 使用yolo进行逐帧物体检测,得到每一帧的检测结果。
2. 将每一帧的检测结果绘制在对应的视频帧上。
3. 将绘制好物体框和标签的视频帧按顺序合成为一个视频。
以下是一个简单的Python代码示例,用于实现yolo逐帧检测后输出视频:
```
import cv2
import numpy as np
import os
# 加载yolo模型
net = cv2.dnn.readNet("yolov3.weights", "yolov3.cfg")
classes = []
with open("coco.names", "r") as f:
classes = [line.strip() for line in f.readlines()]
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 加载视频
cap = cv2.VideoCapture("input_video.mp4")
# 设置输出视频编解码器
fourcc = cv2.VideoWriter_fourcc(*'XVID')
# 获取视频帧率、尺寸等信息
fps = cap.get(cv2.CAP_PROP_FPS)
width = int(cap.get(cv2.CAP_PROP_FRAME_WIDTH))
height = int(cap.get(cv2.CAP_PROP_FRAME_HEIGHT))
# 创建输出视频对象
out = cv2.VideoWriter('output_video.avi', fourcc, fps, (width, height))
# 逐帧检测并输出视频
while True:
ret, frame = cap.read()
if not ret:
break
height, width, channels = frame.shape
# 进行yolo物体检测
blob = cv2.dnn.blobFromImage(frame, 0.00392, (416, 416), (0, 0, 0), True, crop=False)
net.setInput(blob)
outs = net.forward(output_layers)
# 解析检测结果并绘制物体框和标签
class_ids = []
confidences = []
boxes = []
for out in outs:
for detection in out:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * width)
center_y = int(detection[1] * height)
w = int(detection[2] * width)
h = int(detection[3] * height)
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
indexes = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
font = cv2.FONT_HERSHEY_PLAIN
colors = np.random.uniform(0, 255, size=(len(classes), 3))
for i in range(len(boxes)):
if i in indexes:
x, y, w, h = boxes[i]
label = str(classes[class_ids[i]])
color = colors[class_ids[i]]
cv2.rectangle(frame, (x, y), (x + w, y + h), color, 2)
cv2.putText(frame, label, (x, y + 30), font, 3, color, 3)
# 输出视频帧
out.write(frame)
# 释放资源
cap.release()
out.release()
cv2.destroyAllWindows()
```
在上述代码中,我们首先加载yolo模型和视频文件,然后设置输出视频的编解码器和参数。通过逐帧读取视频帧,对每一帧进行yolo物体检测,并将检测结果绘制在对应的视频帧上。最后,将绘制好物体框和标签的视频帧按顺序合成为一个视频,输出到指定文件中。
相关推荐
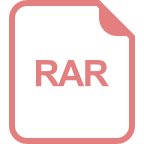












