Overloading and default parameters是什么意思
时间: 2023-05-11 20:02:12 浏览: 63
?Overloading指的是在同一个作用域内,可以定义多个同名的函数或方法,但是它们的参数类型或数量必须不同。而default parameters则是指在函数或方法定义时,可以为某些参数指定默认值,这样在调用时如果没有传入该参数,则会使用默认值。
相关问题
Describe and contrast overloading in Java and Haskell.
Overloading in Java and Haskell are similar in concept but differ in implementation.
In Java, method overloading allows multiple methods to have the same name but with different parameters. The compiler determines which method to call based on the number and type of arguments passed. This allows for more flexibility and readability in code.
For example:
```
public int add(int x, int y) {
return x + y;
}
public double add(double x, double y) {
return x + y;
}
```
In Haskell, function overloading is achieved through the use of type classes. Type classes define a set of functions that can operate on a particular type. This allows for polymorphic behavior and avoids the need for explicit type annotations.
For example:
```
class Addable a where
add :: a -> a -> a
instance Addable Int where
add x y = x + y
instance Addable Double where
add x y = x + y
```
In this example, the `Addable` type class defines the `add` function for types that are instances of the type class. The `instance` keyword is used to define specific implementations of the function for different types.
Overall, overloading in Java and Haskell serve similar purposes but differ in syntax and implementation. Java uses method overloading with different parameter types, while Haskell uses type classes to provide polymorphic behavior.
继承object的类是什么意思
在Python 2中,为了使类成为新式类,需要显示地继承object类。在Python 3中,所有的类都默认继承自object类,因此不再需要显示地继承object类了。
继承object类意味着这个类是一个新式类,具有一些新式类的特性,例如支持属性修饰符(property)、方法重载(method overloading)等。同时,继承自object类还能够保证这个类具有一些通用的方法,例如__str__、__eq__等,这些方法是在object类中定义的。
因此,在Python 3中,如果你不需要显式地继承一个类,可以直接定义一个空类,例如:
```python
class MyClass:
pass
```
这个类会自动继承自object类,具有所有新式类的特性。
相关推荐
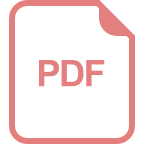
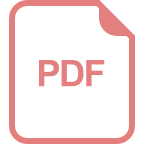
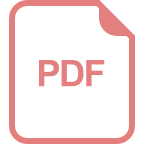












