python众数
时间: 2023-06-21 10:13:50 浏览: 61
求一个列表中的众数可以通过以下代码实现:
```python
from collections import Counter
def mode(numbers):
c = Counter(numbers)
return [k for k, v in c.items() if v == c.most_common(1)[0][1]]
```
其中,使用 `collections` 模块中的 `Counter` 对象来统计列表中每个元素出现的次数,然后找到出现次数最多的元素,最后返回所有出现次数等于最多次数的元素组成的列表。
相关问题
python众数、中位数、平均数
以下是Python求众数、中位数和平均数的示例代码:
1. 平均数:
```python
import numpy as np
a = np.random.randint(0, 10, size=(20)) # 生成一个取值范围为0-10,大小为20的整数数组
average = np.mean(a) # 使用numpy库中的mean函数求平均数
print("平均数:", average)
```
2. 众数:
```python
from scipy import stats
a = np.random.randint(0, 10, size=(20)) # 生成一个取值范围为0-10,大小为20的整数数组
mode = stats.mode(a) # 使用scipy库中的mode函数求众数
print("众数:", mode.mode)
```
3. 中位数:
```python
a = np.random.randint(0, 10, size=(20)) # 生成一个取值范围为0-10,大小为20的整数数组
sorted_a = np.sort(a) # 对数组进行排序
if len(sorted_a) % 2 == 1:
median = sorted_a[int(len(sorted_a) / 2)] # 数组长度为奇数时,中位数为中间的元素
else:
median = (sorted_a[int(len(sorted_a) / 2)] + sorted_a[int(len(sorted_a) / 2) - 1]) / 2 # 数组长度为偶数时,中位数为中间两个元素的平均值
print("中位数:", median)
```
python寻找众数
在Python中,可以使用scipy库的mode函数来寻找众数。该函数返回一个元组,包含众数以及众数出现的次数。例如,对于以下列表[1, 2, 2, 3, 3, 3, 4, 4, 4, 4],可以使用以下代码寻找众数:
```
from scipy import stats
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
mode_result = stats.mode(numbers)
print("众数为:", mode_result.mode)
print("众数出现的次数为:", mode_result.count)
```
输出结果为:
```
众数为: 4
众数出现的次数为: 4
```
相关推荐
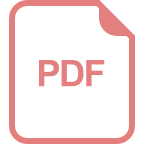
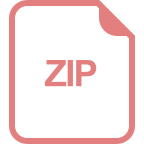












