通过Python实现,输入一行文本,统计其中每个单词的长度。
时间: 2024-02-01 19:15:41 浏览: 80
可以使用Python内置函数`split()`将输入的文本按照空格分割成单词列表,然后遍历列表统计每个单词的长度。
下面是代码示例:
```python
text = input("请输入一行文本:")
words = text.split()
for word in words:
print(f"{word}: {len(word)}")
```
运行示例:
```
请输入一行文本:hello world, this is a text.
hello: 5
world,: 6
this: 4
is: 2
a: 1
text.: 5
```
相关问题
python输入一行文本,统计其中每个单词的长度
您可以使用 Python 的 split() 函数来把输入的文本拆分成单个单词,然后再使用 len() 函数来计算每个单词的长度。以下是一个示例代码:
```python
text = input("请输入一行文本:")
words = text.split() # 使用 split() 函数拆分文本成单个单词
lengths = [len(word) for word in words] # 使用列表解析式计算每个单词的长度
for i in range(len(words)):
print(f"单词 '{words[i]}' 的长度为 {lengths[i]}")
```
示例输出:
```
请输入一行文本:Hello world, this is Python.
单词 'Hello' 的长度为 5
单词 'world,' 的长度为 6
单词 'this' 的长度为 4
单词 'is' 的长度为 2
单词 'Python.' 的长度为 7
```
使用Python,输入一行文本,统计其中每个单词的长度
可以使用Python的内置函数`len()`和字符串方法`split()`来实现。具体步骤如下:
1. 使用`input()`函数获取用户输入的文本
2. 使用字符串方法`split()`将文本分割成单词列表
3. 遍历单词列表,使用内置函数`len()`统计每个单词的长度并输出
下面是示例代码:
```python
text = input("请输入一行文本:")
words = text.split() # 将文本分割成单词列表
for word in words:
print(f"{word} 的长度为 {len(word)}")
```
示例输出:
```
请输入一行文本:Hello world, I am a Python program.
Hello 的长度为 5
world, 的长度为 6
I 的长度为 1
am 的长度为 2
a 的长度为 1
Python 的长度为 6
program. 的长度为 8
```
阅读全文
相关推荐
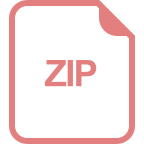
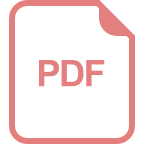

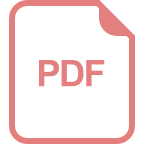
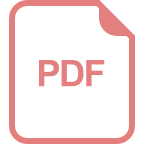
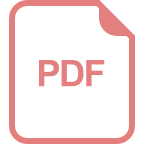
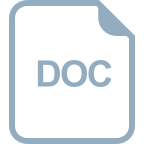








