用c语言编写已知一稀疏矩阵(稀疏矩阵自己定义),写出该稀疏矩阵的三元组顺序表和三元组单链表
时间: 2024-02-06 20:12:15 浏览: 71
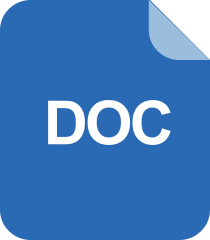
数据结构C语言版 稀疏矩阵的三元组顺序表存储表示和实现.doc
好的,我可以回答你的问题,并提供代码实现。
首先,我们需要定义稀疏矩阵的结构体。
```c
typedef struct {
int row; // 矩阵行数
int col; // 矩阵列数
int num; // 非零元素个数
int **data; // 存储矩阵元素
} Matrix;
```
接下来,我们来实现三元组顺序表。
```c
typedef struct {
int row; // 行下标
int col; // 列下标
int val; // 元素值
} Triple;
typedef struct {
Triple *data; // 存储三元组顺序表的数组
int length; // 非零元素个数
int size; // 数组长度
} TripleList;
void matrixToTripleList(Matrix *matrix, TripleList *list) {
list->length = matrix->num;
list->size = list->length + 1;
list->data = (Triple *)malloc(list->size * sizeof(Triple));
int count = 0;
for (int i = 0; i < matrix->row; i++) {
for (int j = 0; j < matrix->col; j++) {
if (matrix->data[i][j] != 0) {
list->data[count].row = i;
list->data[count].col = j;
list->data[count].val = matrix->data[i][j];
count++;
}
}
}
}
```
接下来,我们来实现三元组单链表。
```c
typedef struct TripleNode {
int row; // 行下标
int col; // 列下标
int val; // 元素值
struct TripleNode *next; // 下一个节点指针
} TripleNode;
typedef struct {
TripleNode *head; // 头结点指针
int length; // 非零元素个数
} TripleLinkedList;
void matrixToTripleLinkedList(Matrix *matrix, TripleLinkedList *list) {
list->head = NULL;
list->length = matrix->num;
TripleNode *last = NULL;
for (int i = 0; i < matrix->row; i++) {
for (int j = 0; j < matrix->col; j++) {
if (matrix->data[i][j] != 0) {
TripleNode *node = (TripleNode *)malloc(sizeof(TripleNode));
node->row = i;
node->col = j;
node->val = matrix->data[i][j];
node->next = NULL;
if (last == NULL) {
list->head = node;
} else {
last->next = node;
}
last = node;
}
}
}
}
```
以上就是稀疏矩阵的三元组顺序表和三元组单链表的实现。
阅读全文
相关推荐





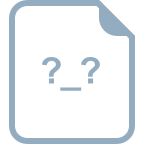
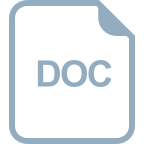
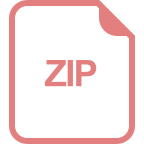
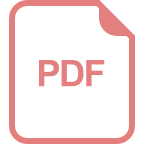
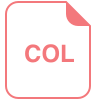
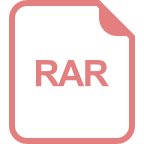