用C#使用简单工厂模式模拟女娲(Nvwa)造人(Person),如果传入参数“M”,则返回一个Man对象,如果传入参数“W”,则返回一个Woman对象。现需要增加一个新的Robot类,如果传入参数“R”,则返回一个Robot对象,对代码进行修改并注意“女娲”的变化。
时间: 2023-12-18 22:05:48 浏览: 87
以下是使用C#实现简单工厂模式模拟女娲造人的代码:
```csharp
using System;
// 人类接口
interface Person
{
void Talk();
}
// 男人类
class Man : Person
{
public void Talk()
{
Console.WriteLine("I am a man.");
}
}
// 女人类
class Woman : Person
{
public void Talk()
{
Console.WriteLine("I am a woman.");
}
}
// 机器人类
class Robot : Person
{
public void Talk()
{
Console.WriteLine("I am a robot.");
}
}
// 简单工厂类
class Nvwa
{
public static Person CreatePerson(string type)
{
switch (type)
{
case "M":
return new Man();
case "W":
return new Woman();
case "R":
return new Robot();
default:
throw new ArgumentException("Invalid type.");
}
}
}
// 测试代码
class Program
{
static void Main(string[] args)
{
Person p1 = Nvwa.CreatePerson("M");
p1.Talk();
Person p2 = Nvwa.CreatePerson("W");
p2.Talk();
Person p3 = Nvwa.CreatePerson("R");
p3.Talk();
// 错误的参数
try
{
Person p4 = Nvwa.CreatePerson("X");
}
catch (ArgumentException ex)
{
Console.WriteLine(ex.Message);
}
}
}
```
在上述代码中,我们定义了一个`Person`接口,并定义了三个实现类`Man`、`Woman`和`Robot`。我们通过`Nvwa`类的静态方法`CreatePerson`来创建不同类型的对象。
测试代码中,我们分别创建了一个男人、一个女人和一个机器人,并调用它们的`Talk`方法输出不同的信息。如果传入的参数无效,将抛出`ArgumentException`异常。
如果需要增加新的类,只需要在`CreatePerson`方法中增加相应的分支即可。
阅读全文
相关推荐
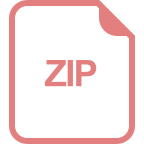



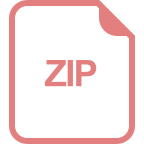
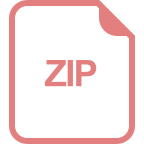
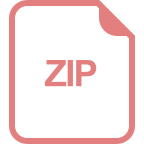
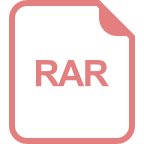
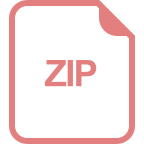
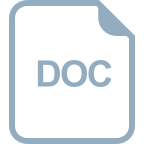


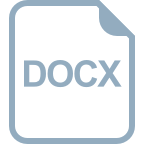