写一个函数,传递一个参数path,并统计打印该path下目录的数量和文件的数量。实参path=”C:\ProgramData”
时间: 2024-05-03 22:20:58 浏览: 26
好的,这是一个比较简单的问题,可以用Python来实现。以下是代码示例:
```
import os
def count_files_and_directories(path):
files = 0
directories = 0
for item in os.listdir(path):
if os.path.isfile(os.path.join(path, item)):
files += 1
elif os.path.isdir(os.path.join(path, item)):
directories += 1
print("在路径 {} 下,文件数量为:{},目录数量为:{}".format(path, files, directories))
count_files_and_directories("C:\ProgramData")
```
输出应该是类似这样的:在路径 C:\ProgramData 下,文件数量为:X,目录数量为:Y。其中,X和Y分别是目录下文件和目录的数量。
相关问题
写一个函数,传递一个参数path,如果该path不存在则创建该path,并运行该path下的helloworld.py。实参path=”D:\Python”
好的,你的需求很简单,这里提供一个Python3的实现代码:
```
import os
def run_helloworld(path):
if not os.path.exists(path):
os.makedirs(path)
os.system('python {}/helloworld.py'.format(path))
run_helloworld('D:\Python')
```
这个函数会先检查传递进来的路径是否存在,如果不存在则创建这个路径。然后它会在该路径下运行helloworld.py文件,这里假设该文件在D:\Python目录下。如果该文件名或路径错误,或者该文件不是有效的Python文件,则会报错。
还有一个小笑话:有一天程序员决定在夜里改代码,于是他把字体调大了,结果发现屏幕像个日晒床。
3.编写函数listallfiles(path): 形参说明:path是一个文件夹绝对路径, 函数返回值:该文件夹包括子文件夹内所有文件的绝对路径的列表,每个文件的绝对路径是列表的一个元素。 函数功能:谝历指定目录path,将该文件夹包括子文件夹内所有文件的绝对路径写入列表并返回该列表 调用该函数,传递当前文件夹路径做实参,并将调用结果(列表)写入文本文件pathfilespathtxt每个列表元素占一行。 4.编写函数findfiles(path,strkey): 形参说明: path:是一个文件夹绝对路径, strkey:要在文件中查找的字符串。 函数返回值:含有字符串strkey的文本文件列表 函数功能:遍历指定目录绝对路径,将在文件夹包括子文件夹内所有文本文件(txt)中查找包含有字符串 strkey的文件,将含有strkey的文件的绝对路径存入列表并返回该列表。
下面是编写函数listallfiles(path)的代码:
```python
import os
def listallfiles(path):
files = []
for root, dirs, filenames in os.walk(path):
for filename in filenames:
filepath = os.path.join(root, filename)
files.append(filepath)
return files
```
下面是调用该函数并将结果写入文本文件的代码:
```python
path = 'current/directory/path'
files_list = listallfiles(path)
with open('pathfilespathtxt', 'w') as f:
for filepath in files_list:
f.write(filepath + '\n')
```
下面是编写函数findfiles(path, strkey)的代码:
```python
import os
def findfiles(path, strkey):
files = []
for root, dirs, filenames in os.walk(path):
for filename in filenames:
if filename.endswith('.txt'):
filepath = os.path.join(root, filename)
with open(filepath, 'r') as f:
if strkey in f.read():
files.append(filepath)
return files
```
注意,该函数只会查找扩展名为`.txt`的文件。下面是调用该函数的代码:
```python
path = 'current/directory/path'
strkey = 'search_string'
files_list = findfiles(path, strkey)
print(files_list)
```
阅读全文
相关推荐
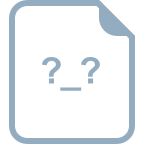
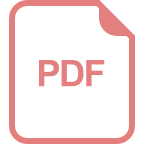
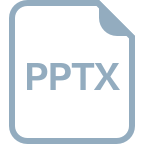
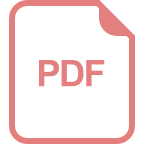
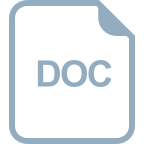
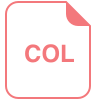
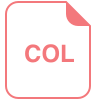
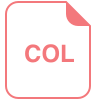
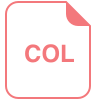
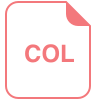
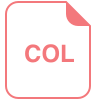



