用python写一个海归交易法
时间: 2023-07-18 16:27:01 浏览: 60
海归交易法是一种基于技术分析的交易策略,利用股票的价格和成交量等数据,通过一定的算法计算出股票的买入和卖出时机,以获得盈利。下面是一个简单的用 Python 实现的海归交易法:
1. 导入必要的库
```python
import pandas as pd
import numpy as np
import talib
import tushare as ts
```
2. 获取股票数据
```python
# 获取股票数据
df = ts.get_k_data('600519', start='2018-01-01', end='2021-01-01')
df.set_index('date', inplace=True)
```
3. 计算指标
```python
# 计算指标
df['ma5'] = talib.MA(df['close'], timeperiod=5)
df['ma10'] = talib.MA(df['close'], timeperiod=10)
df['ma20'] = talib.MA(df['close'], timeperiod=20)
df['ma60'] = talib.MA(df['close'], timeperiod=60)
df['atr'] = talib.ATR(df['high'], df['low'], df['close'], timeperiod=14)
df['rsi'] = talib.RSI(df['close'], timeperiod=14)
df['macd'], df['macdsignal'], df['macdhist'] = talib.MACD(df['close'], fastperiod=12, slowperiod=26, signalperiod=9)
```
4. 制定交易策略
```python
# 制定交易策略
df['signal'] = 0
df['signal'][df['ma5'] > df['ma20']] = 1 # 金叉
df['signal'][df['ma5'] < df['ma20']] = -1 # 死叉
df['position'] = df['signal'].shift(1)
df['position'].fillna(0, inplace=True)
df['stop_loss'] = df['close'] - 2 * df['atr']
df['buy_price'] = np.nan
df['buy_price'][df['position'] == 1] = df['close'][df['position'] == 1]
df['sell_price'] = np.nan
df['sell_price'][df['position'] == -1] = df['close'][df['position'] == -1]
df['buy_price'].fillna(method='ffill', inplace=True)
df['sell_price'].fillna(method='ffill', inplace=True)
df['buy_price'].fillna(0, inplace=True)
df['sell_price'].fillna(0, inplace=True)
df['profit'] = df['sell_price'] - df['buy_price']
df['profit'][df['profit'] < 0] = 0
df['return'] = df['profit'] / df['buy_price'].shift(1)
df['return'][0] = 0
df['cum_return'] = (df['return'] + 1).cumprod()
```
5. 绘制交易曲线
```python
# 绘制交易曲线
import matplotlib.pyplot as plt
plt.plot(df['cum_return'])
plt.xlabel('Date')
plt.ylabel('Cumulative Return')
plt.title('Backtesting of the Trading Strategy')
plt.show()
```
以上代码只是一个简单的海归交易法的实现,实际应用中还需要根据具体情况进行调整和优化。同时,需要注意风险控制的重要性,合理设置止损和止盈等参数。
相关推荐
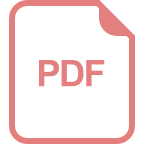
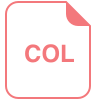
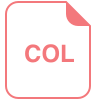
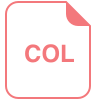
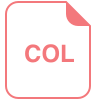
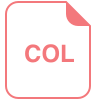






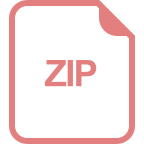
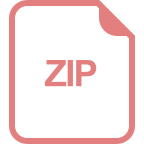
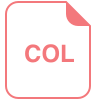