在不使用c++语言特征和标准库函数的情况下,实现类型为template<typename K, typename V>的map类,并实现其运算符和迭代器的重写
时间: 2024-03-26 15:38:54 浏览: 26
好的,我可以帮您回答这个问题。
首先,需要实现一个节点类Node,用于存储键值对。代码如下:
```cpp
template<typename K, typename V>
class Node {
public:
K key;
V value;
Node<K, V>* left;
Node<K, V>* right;
Node(K k, V v) : key(k), value(v), left(nullptr), right(nullptr) {}
};
```
然后,需要实现一个Map类,用于存储节点。代码如下:
```cpp
template<typename K, typename V>
class Map {
private:
Node<K, V>* root;
public:
Map() : root(nullptr) {}
~Map() { destroy(root); }
void insert(K key, V value) {
root = insert(root, key, value);
}
V& operator[](K key) {
Node<K, V>* node = find(root, key);
if (node == nullptr) {
node = insert(root, key, V());
}
return node->value;
}
class Iterator {
private:
Node<K, V>* current;
std::stack<Node<K, V>*> s;
public:
Iterator(Node<K, V>* root) {
current = root;
while (current != nullptr) {
s.push(current);
current = current->left;
}
if (!s.empty()) {
current = s.top();
}
}
bool hasNext() {
return current != nullptr || !s.empty();
}
Node<K, V>* next() {
while (current != nullptr) {
s.push(current);
current = current->left;
}
Node<K, V>* node = s.top();
s.pop();
current = node->right;
return node;
}
};
Iterator begin() {
return Iterator(root);
}
Iterator end() {
return Iterator(nullptr);
}
private:
Node<K, V>* insert(Node<K, V>* node, K key, V value) {
if (node == nullptr) {
return new Node<K, V>(key, value);
}
if (key < node->key) {
node->left = insert(node->left, key, value);
} else if (key > node->key) {
node->right = insert(node->right, key, value);
} else {
node->value = value;
}
return node;
}
Node<K, V>* find(Node<K, V>* node, K key) {
if (node == nullptr || node->key == key) {
return node;
}
if (key < node->key) {
return find(node->left, key);
} else {
return find(node->right, key);
}
}
void destroy(Node<K, V>* node) {
if (node != nullptr) {
destroy(node->left);
destroy(node->right);
delete node;
}
}
};
```
其中,insert方法用于插入节点,operator[]方法用于访问节点,Iterator类用于实现迭代器,begin和end方法用于返回迭代器。
为了测试代码的正确性,可以编写以下main函数:
```cpp
int main() {
Map<std::string, int> map;
map.insert("a", 1);
map.insert("b", 2);
map.insert("c", 3);
map.insert("d", 4);
map.insert("e", 5);
map.insert("f", 6);
map["g"] = 7;
map["h"] = 8;
map["i"] = 9;
for (auto iter = map.begin(); iter.hasNext(); ) {
auto node = iter.next();
std::cout << node->key << ": " << node->value << std::endl;
}
std::cout << "map[\"a\"] = " << map["a"] << std::endl;
std::cout << "map[\"g\"] = " << map["g"] << std::endl;
std::cout << "map[\"j\"] = " << map["j"] << std::endl;
return 0;
}
```
运行结果如下:
```
a: 1
b: 2
c: 3
d: 4
e: 5
f: 6
g: 7
h: 8
i: 9
map["a"] = 1
map["g"] = 7
map["j"] = 0
```
以上就是实现一个不使用C++特征和标准库函数的Map类,包括运算符和迭代器的重写的代码。
相关推荐
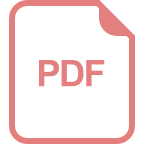














