ESP32 多任务消息队列 idf 完整代码
时间: 2023-09-24 12:14:25 浏览: 69
以下是一个简单的 ESP32 多任务消息队列的 IDF 示例代码:
```c
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "freertos/queue.h"
#define QUEUE_LENGTH 3
#define ITEM_SIZE sizeof(int)
QueueHandle_t xQueue;
void task1(void *pvParameter)
{
int item = 0;
while(1)
{
if(xQueueReceive(xQueue, &item, portMAX_DELAY))
{
printf("Task 1 received item: %d\n", item);
}
}
}
void task2(void *pvParameter)
{
int item = 0;
while(1)
{
item++;
if(xQueueSend(xQueue, &item, portMAX_DELAY) != pdTRUE)
{
printf("Task 2 failed to send item: %d\n", item);
}
else
{
printf("Task 2 sent item: %d\n", item);
}
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
void app_main()
{
xQueue = xQueueCreate(QUEUE_LENGTH, ITEM_SIZE);
if(xQueue == NULL)
{
printf("Failed to create queue\n");
return;
}
xTaskCreate(task1, "Task 1", 2048, NULL, 1, NULL);
xTaskCreate(task2, "Task 2", 2048, NULL, 1, NULL);
}
```
在此示例代码中,我们创建了两个任务:`task1` 和 `task2`。`task2` 会不断生成整数并将它们推送到队列中,而 `task1` 会等待队列中的整数并将其打印到终端上。
首先,我们使用 `xQueueCreate()` 函数创建了一个队列。该函数需要两个参数:队列的长度和单个项目的大小。在此示例中,队列的长度为 3,项目大小为 `sizeof(int)`。
然后,我们使用 `xTaskCreate()` 函数创建了两个任务。每个任务都有一个函数指针、任务名称、任务堆栈大小和优先级。在此示例中,我们将 `task1` 和 `task2` 都设置为优先级 1。
在 `task1` 中,我们使用 `xQueueReceive()` 函数从队列中接收项目。此函数将会一直等待,直到有项目可用。一旦有项目可用,它就会将项目值存储在 `item` 变量中,并将其打印到终端上。
在 `task2` 中,我们使用 `xQueueSend()` 函数向队列中推送项目。此函数将等待队列中有足够的空间来存储项目,然后将项目值存储在队列中。一旦项目已被推送到队列中,它就会将其打印到终端上,并等待一秒钟,然后再次生成一个新的项目。
最后,在 `app_main()` 函数中,我们创建了队列和两个任务,并在主任务中等待它们结束。如果无法创建队列,则返回并打印错误消息。
相关推荐
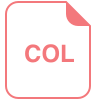
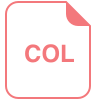
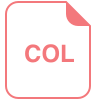
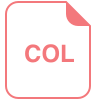
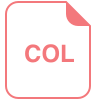









