Java窗口选中表格中的商品,点击结算把窗口表格里的数据以及数据库里数据减掉for判断
时间: 2024-03-07 08:47:43 浏览: 18
假设你的表格是一个 `JTable` 组件,其中包含了商品的信息,而且你的数据库中也存储了商品的信息,那么你可以按照以下步骤来完成你的需求:
1. 获取用户选中的商品行,并从表格中获取商品信息。
```java
JTable table = ... // 获取表格组件
int[] selectedRows = table.getSelectedRows(); // 获取用户选中的行
for (int row : selectedRows) {
// 通过行号获取商品信息
String id = (String) table.getValueAt(row, 0);
String name = (String) table.getValueAt(row, 1);
int quantity = (int) table.getValueAt(row, 2);
double price = (double) table.getValueAt(row, 3);
// TODO: 根据商品信息更新数据库中相应商品的数量和库存
}
```
2. 更新数据库中相应商品的数量和库存。
这里假设你的数据库中有一个 `products` 表,每个商品有一个唯一的编号(`id`)、商品名(`name`)、数量(`quantity`)和价格(`price`)。你可以使用 JDBC 连接数据库,然后执行 SQL 更新语句来更新相应商品的数量和库存。
```java
Connection conn = ... // 获取数据库连接
PreparedStatement ps = null;
ResultSet rs = null;
try {
// 遍历用户选中的商品行,更新数据库中相应商品的数量和库存
for (int row : selectedRows) {
// 通过行号获取商品信息
String id = (String) table.getValueAt(row, 0);
String name = (String) table.getValueAt(row, 1);
int quantity = (int) table.getValueAt(row, 2);
double price = (double) table.getValueAt(row, 3);
// 执行 SQL 更新语句,更新相应商品的数量和库存
String sql = "UPDATE products SET quantity = quantity - ? WHERE id = ?";
ps = conn.prepareStatement(sql);
ps.setInt(1, quantity); // 设置更新的数量
ps.setString(2, id); // 设置更新的商品编号
ps.executeUpdate();
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (ps != null) {
try {
ps.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
这样,当用户选中商品并点击结算按钮时,程序就会从表格中获取商品信息,并根据商品信息更新相应商品的数量和库存。注意,上面的代码只是一个示例,具体的实现可能需要根据你的实际情况进行调整。另外,如果你希望使用 for 循环来实现,可以将上面的代码改写为:
```java
Connection conn = ... // 获取数据库连接
PreparedStatement ps = null;
ResultSet rs = null;
try {
// 遍历表格中的所有商品行
for (int row = 0; row < table.getRowCount(); row++) {
// 判断该行是否被选中
if (table.isRowSelected(row)) {
// 通过行号获取商品信息
String id = (String) table.getValueAt(row, 0);
String name = (String) table.getValueAt(row, 1);
int quantity = (int) table.getValueAt(row, 2);
double price = (double) table.getValueAt(row, 3);
// 执行 SQL 更新语句,更新相应商品的数量和库存
String sql = "UPDATE products SET quantity = quantity - ? WHERE id = ?";
ps = conn.prepareStatement(sql);
ps.setInt(1, quantity); // 设置更新的数量
ps.setString(2, id); // 设置更新的商品编号
ps.executeUpdate();
}
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (ps != null) {
try {
ps.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
这样,程序就会遍历表格中的所有商品行,判断哪些行被选中,然后更新相应商品的数量和库存。
相关推荐
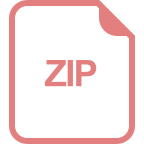
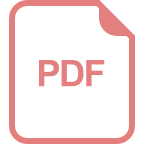
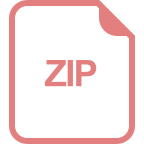














