编写程序,输入字符串s、s的子串s1、字符串s2。要求将s中所有的子串s1替换为s2,输出替换后的字符串。上述字符串中不含空格,替换后字符串的长度不超过100 要求 (1)在函数中进行替换操作,在主函数中输出 (2)使用指针进行操作,字符数组使用动态内存分配管理,不要使用字符串库函数 示例: 输入: abcdeacdfg cd 1234 输出: ab1234ea1234fg 用C语言写
时间: 2024-02-09 20:10:33 浏览: 58
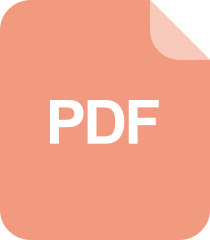
Java获取两个字符串中最大相同子串的方法

可以使用指针和动态内存分配来实现这个字符串替换的功能。具体的实现过程如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *replace(char *s, char *s1, char *s2) {
int len_s = strlen(s);
int len_s1 = strlen(s1);
int len_s2 = strlen(s2);
// 计算新字符串的长度
int count = 0;
char *p = s;
while ((p = strstr(p, s1)) != NULL) {
count++;
p += len_s1;
}
int len_new = len_s + count * (len_s2 - len_s1);
// 分配新字符串的内存
char *new_s = (char *) malloc((len_new + 1) * sizeof(char));
if (new_s == NULL) {
return NULL;
}
// 使用指针实现字符串替换
char *p1 = s;
char *p2 = new_s;
while (*p1 != '\0') {
if (strncmp(p1, s1, len_s1) == 0) {
strncpy(p2, s2, len_s2);
p1 += len_s1;
p2 += len_s2;
} else {
*p2++ = *p1++;
}
}
*p2 = '\0';
return new_s;
}
int main() {
char s[101], s1[101], s2[101];
printf("请输入字符串s:");
scanf("%s", s);
printf("请输入子串s1:");
scanf("%s", s1);
printf("请输入字符串s2:");
scanf("%s", s2);
// 调用替换函数
char *new_s = replace(s, s1, s2);
// 输出结果
if (new_s == NULL) {
printf("内存分配失败!\n");
} else {
printf("替换后的字符串为:%s\n", new_s);
free(new_s);
}
return 0;
}
```
注意,在使用 `malloc()` 动态分配内存时,需要在最后释放内存,避免内存泄漏。另外,由于替换后的字符串长度可能超过原字符串的长度,因此需要动态分配足够的内存。
阅读全文
相关推荐
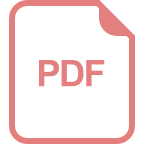
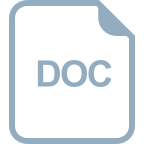












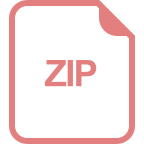
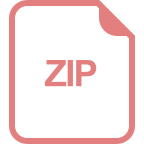