如何用链表写一个车辆管理系统
时间: 2023-08-04 20:10:31 浏览: 98
一个车辆管理系统可以使用链表来实现。每个节点代表一个车辆,包含车辆的信息,如车牌号、品牌、型号等。
以下是一个简单的车辆管理系统的链表实现的示例代码:
```c++
#include <iostream>
#include <string>
using namespace std;
// 车辆节点
struct Vehicle {
string licensePlate; // 车牌号
string brand; // 品牌
string model; // 型号
Vehicle* next; // 下一个节点指针
};
// 车辆管理系统
class VehicleManagement {
private:
Vehicle* head; // 链表头指针
public:
// 构造函数
VehicleManagement() {
head = NULL; // 初始化链表头指针
}
// 添加车辆
void addVehicle(string licensePlate, string brand, string model) {
Vehicle* vehicle = new Vehicle; // 创建新节点
vehicle->licensePlate = licensePlate; // 设置车牌号
vehicle->brand = brand; // 设置品牌
vehicle->model = model; // 设置型号
vehicle->next = head; // 将新节点插入到链表头
head = vehicle; // 更新链表头指针
}
// 删除车辆
void deleteVehicle(string licensePlate) {
if (head == NULL) { // 链表为空
return;
}
if (head->licensePlate == licensePlate) { // 车辆在链表头
Vehicle* temp = head;
head = head->next;
delete temp;
return;
}
Vehicle* current = head->next;
Vehicle* previous = head;
while (current) { // 遍历链表查找车辆
if (current->licensePlate == licensePlate) {
previous->next = current->next;
delete current;
return;
}
previous = current;
current = current->next;
}
}
// 显示所有车辆
void displayAllVehicles() {
if (head == NULL) {
cout << "No vehicles found." << endl;
return;
}
Vehicle* current = head;
while (current) {
cout << "License Plate: " << current->licensePlate << endl;
cout << "Brand: " << current->brand << endl;
cout << "Model: " << current->model << endl;
current = current->next;
}
}
};
int main() {
VehicleManagement vm;
vm.addVehicle("AB1234", "Toyota", "Corolla");
vm.addVehicle("CD5678", "Honda", "Civic");
vm.addVehicle("EF9012", "Ford", "Mustang");
vm.displayAllVehicles();
vm.deleteVehicle("CD5678");
vm.displayAllVehicles();
return 0;
}
```
在这个示例中,我们使用了一个 `Vehicle` 结构体来表示车辆节点。每个节点都包含车牌号、品牌和型号,并且有一个指向下一个节点的指针。
在 `VehicleManagement` 类中,我们实现了三个方法来操作车辆管理系统:
- `addVehicle(string licensePlate, string brand, string model)`:添加一个车辆到链表中。
- `deleteVehicle(string licensePlate)`:删除一个指定车牌号的车辆节点。
- `displayAllVehicles()`:遍历整个链表并输出每个车辆的信息。
在 `main` 函数中,我们创建了一个 `VehicleManagement` 对象并添加了三个车辆到链表中。然后我们调用了 `displayAllVehicles` 方法来显示所有车辆,并删除了一个车辆节点。最后,我们再次调用 `displayAllVehicles` 方法来验证车辆是否正确地被删除。
阅读全文
相关推荐
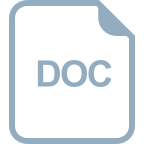



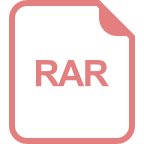
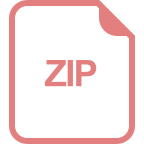
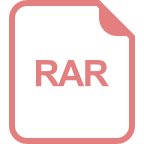
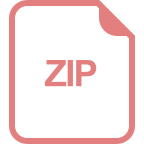
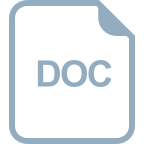
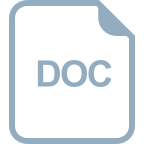
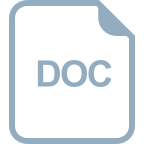




