谷歌小恐龙c++代码
时间: 2023-08-06 17:16:02 浏览: 307
以下是谷歌小恐龙的简单实现代码(使用C++和SDL库):
```c++
#include <iostream>
#include <SDL.h>
using namespace std;
const int SCREEN_WIDTH = 600;
const int SCREEN_HEIGHT = 200;
const int GROUND_HEIGHT = 180;
int main(int argc, char* args[])
{
SDL_Init(SDL_INIT_VIDEO);
SDL_Window* window = SDL_CreateWindow("Google Dino", SDL_WINDOWPOS_UNDEFINED, SDL_WINDOWPOS_UNDEFINED, SCREEN_WIDTH, SCREEN_HEIGHT, SDL_WINDOW_SHOWN);
SDL_Renderer* renderer = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED);
SDL_Texture* dinoTexture = SDL_CreateTextureFromSurface(renderer, SDL_LoadBMP("dino.bmp"));
SDL_Texture* cactusTexture = SDL_CreateTextureFromSurface(renderer, SDL_LoadBMP("cactus.bmp"));
SDL_Texture* groundTexture = SDL_CreateTextureFromSurface(renderer, SDL_LoadBMP("ground.bmp"));
bool quit = false;
SDL_Event e;
int dinoX = 0;
int dinoY = GROUND_HEIGHT;
int cactusX = SCREEN_WIDTH;
int cactusY = GROUND_HEIGHT - 20;
int groundX = 0;
int groundY = GROUND_HEIGHT + 20;
int dinoVelY = 0;
while (!quit)
{
while (SDL_PollEvent(&e) != 0)
{
if (e.type == SDL_QUIT)
{
quit = true;
}
else if (e.type == SDL_KEYDOWN)
{
if (e.key.keysym.sym == SDLK_SPACE)
{
if (dinoY == GROUND_HEIGHT)
{
dinoVelY = -10;
}
}
}
}
dinoY += dinoVelY;
if (dinoY > GROUND_HEIGHT)
{
dinoY = GROUND_HEIGHT;
dinoVelY = 0;
}
else if (dinoY < 0)
{
dinoY = 0;
dinoVelY = 0;
}
cactusX -= 5;
if (cactusX < -20)
{
cactusX = SCREEN_WIDTH;
}
groundX -= 5;
if (groundX < -SCREEN_WIDTH)
{
groundX = 0;
}
SDL_SetRenderDrawColor(renderer, 255, 255, 255, 255);
SDL_RenderClear(renderer);
SDL_Rect dinoRect = { dinoX, dinoY, 48, 48 };
SDL_RenderCopy(renderer, dinoTexture, NULL, &dinoRect);
SDL_Rect cactusRect = { cactusX, cactusY, 20, 40 };
SDL_RenderCopy(renderer, cactusTexture, NULL, &cactusRect);
SDL_Rect groundRect1 = { groundX, groundY, SCREEN_WIDTH, 20 };
SDL_RenderCopy(renderer, groundTexture, NULL, &groundRect1);
SDL_Rect groundRect2 = { groundX + SCREEN_WIDTH, groundY, SCREEN_WIDTH, 20 };
SDL_RenderCopy(renderer, groundTexture, NULL, &groundRect2);
SDL_RenderPresent(renderer);
SDL_Delay(17);
}
SDL_DestroyTexture(dinoTexture);
SDL_DestroyTexture(cactusTexture);
SDL_DestroyTexture(groundTexture);
SDL_DestroyRenderer(renderer);
SDL_DestroyWindow(window);
SDL_Quit();
return 0;
}
```
这只是一个简单的实现,你可以根据需要进行修改和扩展。
相关推荐
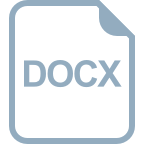














