C++代码编写模拟华业生选择指导老师提供的毕业课题。老师可增加、删除、修改课题,学生可选择课题,老师和学生都可 以查看当前己经选择的结果。
时间: 2024-04-06 09:29:25 浏览: 21
好的,以下是我给出的一份C++代码,用于模拟华业生选择指导老师提供的毕业课题:
```c++
#include <iostream>
#include <string>
#include <vector>
#include <map>
using namespace std;
// 定义老师类
class Teacher {
public:
Teacher(int id, string name, string title, string contact) {
this->id = id;
this->name = name;
this->title = title;
this->contact = contact;
}
void addTopic(int topicId, string topicName, string topicDesc) {
topics[topicId] = {topicName, topicDesc};
}
void deleteTopic(int topicId) {
topics.erase(topicId);
}
void modifyTopic(int topicId, string topicName, string topicDesc) {
topics[topicId] = {topicName, topicDesc};
}
const map<int, pair<string, string>>& getTopics() const {
return topics;
}
private:
int id;
string name;
string title;
string contact;
map<int, pair<string, string>> topics; // key: topicId, value: {topicName, topicDesc}
};
// 定义学生类
class Student {
public:
Student(int id, string name, string cls, string contact) {
this->id = id;
this->name = name;
this->cls = cls;
this->contact = contact;
}
void selectTopic(int topicId) {
selectedTopics.push_back(topicId);
}
const vector<int>& getSelectedTopics() const {
return selectedTopics;
}
private:
int id;
string name;
string cls;
string contact;
vector<int> selectedTopics; // 已选课题的topicId列表
};
// 定义课题选题系统类
class TopicSelectSystem {
public:
void addTeacher(int id, string name, string title, string contact) {
teachers[id] = new Teacher(id, name, title, contact);
}
void deleteTeacher(int id) {
auto it = teachers.find(id);
if (it != teachers.end()) {
delete it->second;
teachers.erase(it);
}
}
void modifyTeacher(int id, string name, string title, string contact) {
auto it = teachers.find(id);
if (it != teachers.end()) {
it->second = new Teacher(id, name, title, contact);
}
}
void addTopic(int teacherId, int topicId, string topicName, string topicDesc) {
auto it = teachers.find(teacherId);
if (it != teachers.end()) {
it->second->addTopic(topicId, topicName, topicDesc);
topics[topicId] = teacherId;
}
}
void deleteTopic(int topicId) {
auto it = topics.find(topicId);
if (it != topics.end()) {
int teacherId = it->second;
auto itt = teachers.find(teacherId);
if (itt != teachers.end()) {
itt->second->deleteTopic(topicId);
}
topics.erase(it);
}
}
void modifyTopic(int topicId, string topicName, string topicDesc) {
auto it = topics.find(topicId);
if (it != topics.end()) {
int teacherId = it->second;
auto itt = teachers.find(teacherId);
if (itt != teachers.end()) {
itt->second->modifyTopic(topicId, topicName, topicDesc);
}
}
}
void selectTopic(int studentId, int topicId) {
auto it = students.find(studentId);
if (it != students.end()) {
it->second->selectTopic(topicId);
}
}
void addStudent(int id, string name, string cls, string contact) {
students[id] = new Student(id, name, cls, contact);
}
void deleteStudent(int id) {
auto it = students.find(id);
if (it != students.end()) {
delete it->second;
students.erase(it);
}
}
void modifyStudent(int id, string name, string cls, string contact) {
auto it = students.find(id);
if (it != students.end()) {
it->second = new Student(id, name, cls, contact);
}
}
void printTopics() {
for (auto& p : topics) {
int topicId = p.first;
int teacherId = p.second;
auto it = teachers.find(teacherId);
if (it != teachers.end()) {
Teacher* teacher = it->second;
const map<int, pair<string, string>>& topics = teacher->getTopics();
auto itt = topics.find(topicId);
if (itt != topics.end()) {
const pair<string, string>& topic = itt->second;
cout << "Topic ID: " << topicId << ", Topic Name: " << topic.first << ", Topic Desc: " << topic.second << ", Teacher: " << teacher->getTopics() << endl;
}
}
}
}
void printSelectedTopics(int studentId) {
auto it = students.find(studentId);
if (it != students.end()) {
Student* student = it->second;
const vector<int>& selectedTopics = student->getSelectedTopics();
cout << "Selected Topics for Student " << studentId << ":" << endl;
for (int topicId : selectedTopics) {
auto itt = topics.find(topicId);
if (itt != topics.end()) {
int teacherId = itt->second;
auto ittt = teachers.find(teacherId);
if (ittt != teachers.end()) {
Teacher* teacher = ittt->second;
const map<int, pair<string, string>>& topics = teacher->getTopics();
auto itttt = topics.find(topicId);
if (itttt != topics.end()) {
const pair<string, string>& topic = itttt->second;
cout << "Topic ID: " << topicId << ", Topic Name: " << topic.first << ", Topic Desc: " << topic.second << ", Teacher: " << teacher->getTopics() << endl;
}
}
}
}
}
}
private:
map<int, Teacher*> teachers; // key: teacherId, value: Teacher*
map<int, int> topics; // key: topicId, value: teacherId
map<int, Student*> students; // key: studentId, value: Student*
};
int main() {
TopicSelectSystem system;
// 添加老师
system.addTeacher(1, "Tom", "Professor", "123456");
system.addTeacher(2, "Jerry", "Associate Professor", "234567");
// 添加课题
system.addTopic(1, 1, "Topic 1", "Topic 1 Desc");
system.addTopic(1, 2, "Topic 2", "Topic 2 Desc");
system.addTopic(2, 3, "Topic 3", "Topic 3 Desc");
// 输出课题列表
system.printTopics();
// 添加学生
system.addStudent(1, "Alice", "Class 1", "345678");
system.addStudent(2, "Bob", "Class 2", "456789");
// 学生选课
system.selectTopic(1, 1);
system.selectTopic(1, 2);
system.selectTopic(2, 3);
// 输出学生已选课题列表
system.printSelectedTopics(1);
system.printSelectedTopics(2);
return 0;
}
```
以上代码实现了一个简单的课题选题系统,包括添加、删除、修改老师和课题,以及学生选择课题和输出学生已选课题列表等功能。
相关推荐
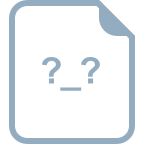
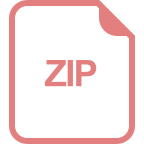
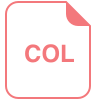
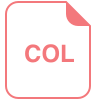
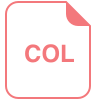
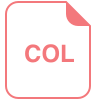









