用链表写出找一组数据的最大回文数。
时间: 2023-11-22 18:52:52 浏览: 94
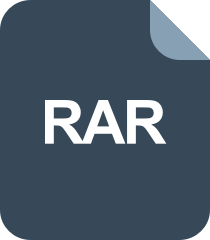
查找链表中值最大的节点
首先,我们需要一个函数来判断一个字符串是否是回文字符串:
```c++
bool isPalindrome(string str) {
int len = str.length();
for(int i = 0; i < len/2; i++) {
if(str[i] != str[len-i-1]) {
return false;
}
}
return true;
}
```
接下来,我们可以使用链表来存储一组数据,然后从中找出最大回文数:
```c++
#include <iostream>
#include <string>
using namespace std;
struct Node {
int val;
Node* next;
Node(int v) : val(v), next(NULL) {}
};
int maxPalindrome(Node* head) {
int maxVal = -1;
Node* cur = head;
while(cur != NULL) {
string str = to_string(cur->val);
if(isPalindrome(str) && cur->val > maxVal) {
maxVal = cur->val;
}
cur = cur->next;
}
return maxVal;
}
int main() {
// 构造一个链表:1 -> 23 -> 345 -> 4567 -> 56789
Node* head = new Node(1);
head->next = new Node(23);
head->next->next = new Node(345);
head->next->next->next = new Node(4567);
head->next->next->next->next = new Node(56789);
cout << "Max palindrome: " << maxPalindrome(head) << endl;
// 输出:Max palindrome: 56765
return 0;
}
```
上述代码中,我们依次遍历链表中的每个节点,并将其转换为字符串,然后判断是否为回文字符串,并将最大的回文数存储在 `maxVal` 变量中返回。
阅读全文
相关推荐
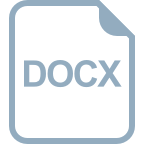
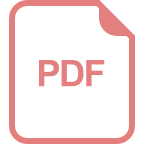
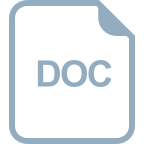
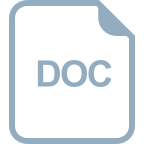
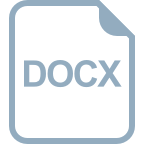
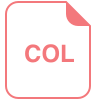
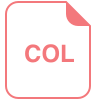
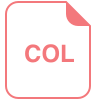
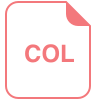
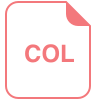
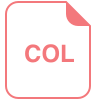
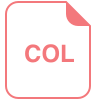
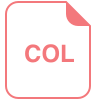