int number; Student** students; void student()//录入学生信息 { cout << "请输入你要录入的人数:" << endl; int number; cin >> number; students = new Student * [number]; string name;//姓名 int age;//年龄 string id;//学号 double score; ofstream outfile; outfile.open("D:\\sdata.txt", ios::out); for (int i = 0; i < number; i++) { system("cls"); cout << "请依次输入学生的id,姓名,年龄,得分" << endl; cin >> id >> name >> age >> score; Student a(id, name, age, score); students[i] = &a; outfile << "Id" << " " << "Name" << " " << "Age" << " " << "Score" << endl; outfile << students[i]->getId() << " " << students[i]->getName() << " " << students[i] ->getAge() <<" " << students[i]->getScore() << endl; } for (int i = 0; i < number; i++) { delete students[i]; }delete[] students; outfile.close(); }检查错误
时间: 2024-01-25 10:02:49 浏览: 28
这段代码有几个问题:
1. 函数名和变量名重复了,不应该将函数命名为 `student()`,这可能会导致错误。应该考虑将其命名为 `input_students()` 或者其他不与变量名冲突的名称。
2. 在 `input_students()` 函数内部,你声明了一个局部变量 `number`,这个变量会隐藏掉函数外部的全局变量 `int number;`,导致在函数结束后无法访问全局变量 `number`。你应该避免这种变量名重复的情况。
3. 在循环中,你使用了 `Student a(id, name, age, score);` 创建了一个 `Student` 类型的对象 `a`,并将其地址赋给了 `students[i]`。但是,在循环结束后,`students` 数组中存储的都是指向 `a` 对象的指针,这是不正确的。因为 `a` 对象的生命周期是在循环结束后结束的,这些指针就变成了悬空指针,访问它们会导致未定义行为。你应该使用 `new` 运算符为每个学生对象分配内存,并将其地址存储在 `students` 数组中。
4. 在循环结束后,你使用了 `delete students[i];` 和 `delete[] students;` 来释放内存。但是,在循环中你使用了 `&a` 将 `a` 的地址存储在了 `students` 数组中,这是不正确的,因为 `a` 是在循环内部定义的局部变量,在循环结束后,它的地址已经失效了,所以不能使用 `delete` 释放它的内存。你应该在循环中使用 `new` 运算符为每个学生对象分配内存,在循环结束后使用 `delete` 释放每个学生对象的内存,最后使用 `delete[]` 释放 `students` 数组的内存。
5. 在循环中,你每次都打开文件 `D:\\sdata.txt`,写入一行数据,然后关闭文件。这样做的效率很低,而且每次打开文件都会覆盖之前的数据。你应该在循环外部打开文件,循环内部写入数据,循环结束后关闭文件。这样可以提高效率,并保留所有的数据。
下面是修改后的代码:
```
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
class Student {
public:
Student(string id, string name, int age, double score) {
this->id = id;
this->name = name;
this->age = age;
this->score = score;
}
string getId() { return id; }
string getName() { return name; }
int getAge() { return age; }
double getScore() { return score; }
private:
string id;
string name;
int age;
double score;
};
void input_students() {
int n;
cout << "请输入你要录入的人数:" << endl;
cin >> n;
Student** students = new Student * [n];
string name;
int age;
string id;
double score;
ofstream outfile("D:\\sdata.txt", ios::out);
for (int i = 0; i < n; i++) {
system("cls");
cout << "请依次输入学生的id,姓名,年龄,得分" << endl;
cin >> id >> name >> age >> score;
Student* s = new Student(id, name, age, score);
students[i] = s;
outfile << s->getId() << " " << s->getName() << " " << s->getAge() << " " << s->getScore() << endl;
}
outfile.close();
for (int i = 0; i < n; i++) {
delete students[i];
}
delete[] students;
}
int main() {
input_students();
return 0;
}
```
相关推荐
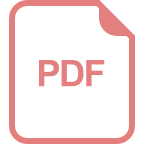
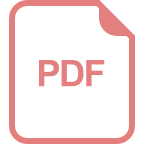
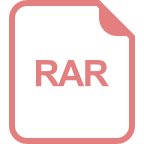















