3. 一年一班共有学生n名,编程实现对该班学生成绩的管理: 数据项:学号、姓名、语文、数学 功能: 录入、查询、统计
时间: 2023-07-10 08:20:10 浏览: 96
以下是一个简单的 C++ 实现,包括学生信息的定义、录入、查询和统计功能:
```cpp
#include <iostream>
#include <string>
using namespace std;
const int MAX_NUM = 100; // 最大学生数
// 学生信息结构体
struct Student {
string id; // 学号
string name; // 姓名
int chinese; // 语文成绩
int math; // 数学成绩
};
// 录入学生信息
void input(Student students[], int& count) {
cout << "请输入学生信息(学号 姓名 语文成绩 数学成绩):" << endl;
while (count < MAX_NUM) {
Student s;
cin >> s.id >> s.name >> s.chinese >> s.math;
if (s.id == "0") { // 学号为0表示录入结束
break;
}
students[count++] = s;
}
}
// 查询学生信息
void search(Student students[], int count) {
string id;
cout << "请输入学号查询学生信息:" << endl;
cin >> id;
for (int i = 0; i < count; i++) {
if (students[i].id == id) {
cout << "姓名:" << students[i].name << endl;
cout << "语文成绩:" << students[i].chinese << endl;
cout << "数学成绩:" << students[i].math << endl;
return;
}
}
cout << "未找到该学生" << endl;
}
// 统计学生成绩
void statistics(Student students[], int count) {
int chinese_sum = 0, math_sum = 0;
for (int i = 0; i < count; i++) {
chinese_sum += students[i].chinese;
math_sum += students[i].math;
}
cout << "语文总分:" << chinese_sum << endl;
cout << "数学总分:" << math_sum << endl;
cout << "平均语文成绩:" << static_cast<double>(chinese_sum) / count << endl;
cout << "平均数学成绩:" << static_cast<double>(math_sum) / count << endl;
}
int main() {
Student students[MAX_NUM];
int count = 0; // 学生数
int choice; // 菜单选项
do {
cout << "请选择操作:" << endl;
cout << "1. 录入学生信息" << endl;
cout << "2. 查询学生信息" << endl;
cout << "3. 统计学生成绩" << endl;
cout << "0. 退出" << endl;
cin >> choice;
switch (choice) {
case 1:
input(students, count);
break;
case 2:
search(students, count);
break;
case 3:
statistics(students, count);
break;
case 0:
break;
default:
cout << "无效选择" << endl;
break;
}
} while (choice != 0);
return 0;
}
```
在录入时,输入学号为 0 表示录入结束。在查询时,输入要查询的学号,如果找到了对应的学生,就输出该学生的姓名、语文成绩和数学成绩;如果没有找到,就输出未找到该学生。在统计时,分别计算所有学生的语文总分、数学总分和平均成绩。
相关推荐
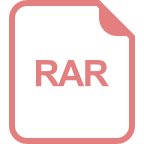
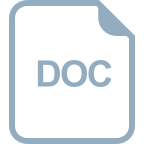














