试编写在顺序表上实现插入和删除的算法。 假设有两个按数据元素值递增有序排列的顺序表A和B,编写算法将A表和B表归并成一个按元素值递增有序排列的顺序表C。 一年一班共有学生n名,编程实现对该班学生成绩的管理: 数据项:学号、姓名、语文、数学 功能: 录入、查询、统计
时间: 2024-02-25 15:58:07 浏览: 32
1. 顺序表插入和删除算法:
插入算法:
```
void insert(int* arr, int pos, int val, int& len) {
if (pos < 0 || pos > len) { // 判断插入位置是否合法
cout << "插入位置不合法!" << endl;
return;
}
for (int i = len - 1; i >= pos; i--) { // 从尾部开始往后移动元素
arr[i + 1] = arr[i];
}
arr[pos] = val; // 插入元素
len++; // 长度加1
}
删除算法:
void remove(int* arr, int pos, int& len) {
if (pos < 0 || pos >= len) { // 判断删除位置是否合法
cout << "删除位置不合法!" << endl;
return;
}
for (int i = pos; i < len - 1; i++) { // 从删除位置开始往前移动元素
arr[i] = arr[i + 1];
}
len--; // 长度减1
}
```
2. 顺序表归并算法:
```
void merge(int* arrA, int lenA, int* arrB, int lenB, int* arrC, int& lenC) {
int i = 0, j = 0, k = 0;
while (i < lenA && j < lenB) { // A表和B表都没有遍历完
if (arrA[i] <= arrB[j]) {
arrC[k++] = arrA[i++];
} else {
arrC[k++] = arrB[j++];
}
}
while (i < lenA) { // A表还有剩余元素
arrC[k++] = arrA[i++];
}
while (j < lenB) { // B表还有剩余元素
arrC[k++] = arrB[j++];
}
lenC = lenA + lenB; // 归并后的长度
}
```
3. 学生成绩管理系统:
定义学生结构体:
```
struct Student {
int id; // 学号
string name; // 姓名
int chinese; // 语文成绩
int math; // 数学成绩
};
```
录入学生成绩:
```
void addStudent(Student* arr, int& len) {
Student s;
cout << "请输入学号:";
cin >> s.id;
cout << "请输入姓名:";
cin >> s.name;
cout << "请输入语文成绩:";
cin >> s.chinese;
cout << "请输入数学成绩:";
cin >> s.math;
arr[len++] = s;
}
```
查询学生成绩:
```
void queryStudent(Student* arr, int len) {
int id;
cout << "请输入要查询的学号:";
cin >> id;
for (int i = 0; i < len; i++) {
if (arr[i].id == id) {
cout << "学号:" << arr[i].id << endl;
cout << "姓名:" << arr[i].name << endl;
cout << "语文成绩:" << arr[i].chinese << endl;
cout << "数学成绩:" << arr[i].math << endl;
return;
}
}
cout << "没有找到该学生信息!" << endl;
}
```
统计学生成绩:
```
void countStudent(Student* arr, int len) {
int chineseTotal = 0, mathTotal = 0;
for (int i = 0; i < len; i++) {
chineseTotal += arr[i].chinese;
mathTotal += arr[i].math;
}
cout << "语文成绩总分:" << chineseTotal << endl;
cout << "数学成绩总分:" << mathTotal << endl;
cout << "语文成绩平均分:" << chineseTotal * 1.0 / len << endl;
cout << "数学成绩平均分:" << mathTotal * 1.0 / len << endl;
}
```
相关推荐
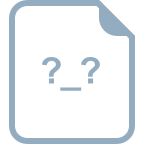
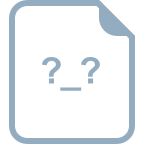
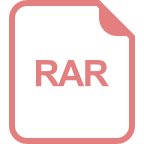













