利用信号量 实现 生产者-消费者问题。
时间: 2024-05-15 15:17:47 浏览: 4
生产者-消费者问题是指一个共享的缓冲区间,生产者将对象放入该缓冲区间,消费者则将对象从缓冲区间取出。但是生产者和消费者不能同时对缓冲区间进行操作,也就是说缓冲区间需要实现互斥访问。而信号量可以用来控制对缓冲区间的访问,实现互斥。
具体地,在实现过程中需要定义两个信号量,一个表示缓冲区间已有的对象数目,另一个表示缓冲区间空的对象数目。生产者在向缓冲区放置对象时,需要先使用空对象信号量进行等待,直到缓冲区间还存在空对象,然后使用已有对象信号量进行加锁,放入对象,释放已有对象信号量。消费者在从缓冲区取出对象时,需要先使用已有对象信号量进行等待,直到缓冲区间有可用的对象,然后使用空对象信号量进行加锁,取出对象,释放空对象信号量。
下面给出一个简单的代码实现:
```
#include <pthread.h>
#include <semaphore.h>
#define MAX_ITEM 10
#define BUFFER_SIZE (MAX_ITEM + 1)
int buffer[BUFFER_SIZE];
int in, out;
sem_t empty, full, mutex;
void *producer(void *arg)
{
int item;
while (1) {
/* produce an item */
item = ...
/* wait for an empty slot */
sem_wait(&empty);
/* lock the buffer */
sem_wait(&mutex);
/* insert the item into the buffer */
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
/* unlock the buffer */
sem_post(&mutex);
/* signal a full slot */
sem_post(&full);
}
}
void *consumer(void *arg)
{
int item;
while (1) {
/* wait for a full slot */
sem_wait(&full);
/* lock the buffer */
sem_wait(&mutex);
/* remove an item from the buffer */
item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
/* unlock the buffer */
sem_post(&mutex);
/* signal an empty slot */
sem_post(&empty);
/* consume the item */
...
}
}
int main()
{
pthread_t tid1, tid2;
/* initialize the buffer */
in = out = 0;
/* initialize the semaphores */
sem_init(&empty, 0, BUFFER_SIZE - 1);
sem_init(&full, 0, 0);
sem_init(&mutex, 0, 1);
/* create the producer and consumer threads */
pthread_create(&tid1, NULL, producer, NULL);
pthread_create(&tid2, NULL, consumer, NULL);
/* wait for the threads to finish */
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
/* destroy the semaphores */
sem_destroy(&empty);
sem_destroy(&full);
sem_destroy(&mutex);
return 0;
}
```
相关推荐
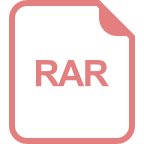
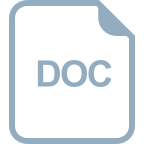
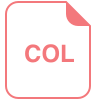
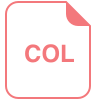
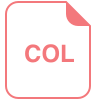
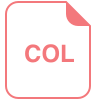
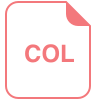









