C#量表实现word文档打印及预览
时间: 2023-08-09 22:11:37 浏览: 206
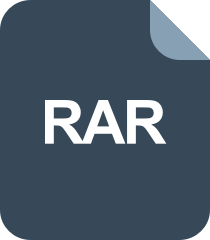
C#实现打印和打印预览功能

在C#中,可以使用Microsoft.Office.Interop.Word和Windows Forms库来实现从用户输入的量表数据生成Word文档的打印和预览。下面是一个简单的示例代码,可以从Windows Forms中读取用户输入的量表数据,将数据插入到Word文档中,然后预览并打印出来:
```csharp
using Microsoft.Office.Interop.Word;
using System.Windows.Forms;
// 声明Word应用程序对象
Application wordApp = new Application();
Document wordDoc = null;
try
{
// 创建一个新的Word文档
wordDoc = wordApp.Documents.Add();
// 插入量表标题
Paragraph titlePara = wordDoc.Content.Paragraphs.Add();
titlePara.Range.Text = "量表标题";
titlePara.Range.Font.Bold = 1;
titlePara.Format.Alignment = WdParagraphAlignment.wdAlignParagraphCenter;
titlePara.Range.InsertParagraphAfter();
// 插入量表数据表格
Table dataTbl = wordDoc.Tables.Add(wordDoc.Content.Paragraphs.Last.Range, 6, 2);
dataTbl.Borders.InsideLineStyle = WdLineStyle.wdLineStyleSingle;
dataTbl.Borders.OutsideLineStyle = WdLineStyle.wdLineStyleSingle;
dataTbl.Cell(1, 1).Range.Text = "题目";
dataTbl.Cell(1, 2).Range.Text = "得分";
// 将用户输入的量表数据插入到表格中
for (int i = 0; i < 5; i++)
{
dataTbl.Cell(i + 2, 1).Range.Text = "第" + (i + 1) + "题";
dataTbl.Cell(i + 2, 2).Range.Text = textBoxes[i].Text; // textBoxes为Windows Forms中的文本框数组
}
// 插入量表总分
Paragraph scorePara = wordDoc.Content.Paragraphs.Add();
scorePara.Range.Text = "总分:" + totalScore;
scorePara.Range.Font.Bold = 1;
scorePara.Format.Alignment = WdParagraphAlignment.wdAlignParagraphRight;
scorePara.Range.InsertParagraphAfter();
// 预览Word文档
wordDoc.PrintPreview();
// 打印Word文档
wordDoc.PrintOut();
// 关闭Word文档
wordDoc.Close(false);
}
catch (Exception ex)
{
Console.WriteLine("Error: " + ex.Message);
}
finally
{
// 关闭Word应用程序
wordApp.Quit(false);
}
```
注意:在使用Windows Forms库时,你需要在项目中添加Windows Forms控件并将其与代码关联。在上面的示例代码中,我们使用了一个文本框数组textBoxes来存储用户输入的量表数据。你需要根据你的具体情况修改代码。
阅读全文
相关推荐
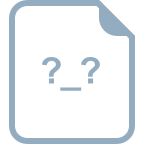
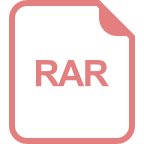
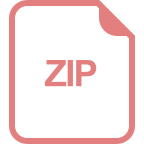
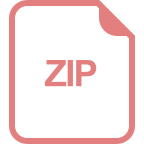
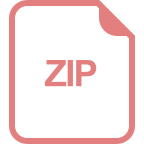
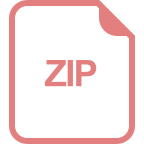
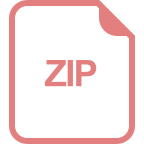
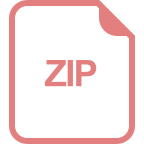
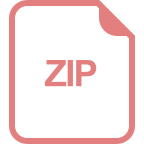
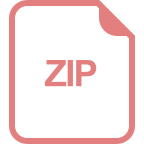
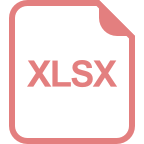
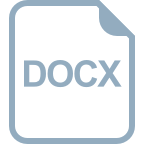
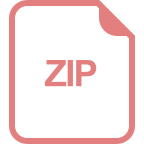