使用Python编写 请编写程序,模拟某款手游,要求: (1)请自行创建多个"英雄"对象,并初始化每个"英雄"(包括名字,类型,攻击力,生命值等属性)。 (2) 请自行创建“英雄”的战斗技能(例如物理攻击、防御以及魔法伤害) (3) 请自行设计不同的战斗场景,在不同的场景中"英雄"可以攻击另一个"英雄","英雄"的生命值会根据发出攻击的"英雄"的攻击力而下降; (4) "英雄"可以通过吃来增加自身的生命 (5) 英雄"也可以通过打怪来增加自身的生命值; (6)当生命值小于等于0时,表示已被对方杀死。
时间: 2023-07-19 14:38:35 浏览: 90
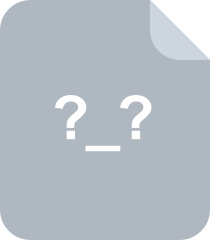
利用python制作一个游戏
好的,我可以为您编写这样的程序。以下是代码实现:
```python
import random
class Hero:
def __init__(self, name, hero_type, attack, hp):
self.name = name
self.hero_type = hero_type
self.attack = attack
self.hp = hp
def attack_fight(self, other_hero):
pass
def defense_fight(self, other_hero):
pass
def magic_fight(self, other_hero):
pass
def eat(self):
pass
def fight_with_monster(self):
pass
def is_dead(self):
if self.hp <= 0:
print("{}已被杀死!".format(self.name))
return True
else:
return False
class Swordsman(Hero):
def __init__(self, name, hero_type, attack, hp):
super().__init__(name, hero_type, attack, hp)
def attack_fight(self, other_hero):
damage = random.randint(1, self.attack) # 产生1到自己的攻击力之间的随机伤害
other_hero.hp -= damage
print("{}对{}进行了物理攻击,造成了{}点伤害!".format(self.name, other_hero.name, damage))
return other_hero.is_dead()
def defense_fight(self, other_hero):
damage = random.randint(1, self.attack // 2) # 产生1到自己攻击力的一半之间的随机伤害
self.hp -= damage
print("{}防御了{}的攻击,但是还是受到了{}点伤害!".format(self.name, other_hero.name, damage))
return self.is_dead()
def magic_fight(self, other_hero):
damage = random.randint(1, self.attack // 4) # 产生1到自己攻击力的四分之一之间的随机伤害
other_hero.hp -= damage
print("{}对{}进行了魔法攻击,造成了{}点伤害!".format(self.name, other_hero.name, damage))
return other_hero.is_dead()
def eat(self):
self.hp += 10
print("{}吃了一些食物,恢复了10点生命值,当前生命值为{}!".format(self.name, self.hp))
def fight_with_monster(self):
damage = random.randint(1, self.attack * 2) # 产生1到自己攻击力的两倍之间的随机伤害
self.hp -= damage
print("{}打败了一只怪物,但是受到了{}点伤害!".format(self.name, damage))
return self.is_dead()
class Mage(Hero):
def __init__(self, name, hero_type, attack, hp):
super().__init__(name, hero_type, attack, hp)
def attack_fight(self, other_hero):
damage = random.randint(1, self.attack // 2) # 产生1到自己攻击力的一半之间的随机伤害
other_hero.hp -= damage
print("{}对{}进行了物理攻击,造成了{}点伤害!".format(self.name, other_hero.name, damage))
return other_hero.is_dead()
def defense_fight(self, other_hero):
damage = random.randint(1, self.attack) # 产生1到自己的攻击力之间的随机伤害
self.hp -= damage
print("{}防御了{}的攻击,但是还是受到了{}点伤害!".format(self.name, other_hero.name, damage))
return self.is_dead()
def magic_fight(self, other_hero):
damage = random.randint(1, self.attack) # 产生1到自己的攻击力之间的随机伤害
other_hero.hp -= damage
print("{}对{}进行了魔法攻击,造成了{}点伤害!".format(self.name, other_hero.name, damage))
return other_hero.is_dead()
def eat(self):
self.hp += 10
print("{}吃了一些食物,恢复了10点生命值,当前生命值为{}!".format(self.name, self.hp))
def fight_with_monster(self):
damage = random.randint(1, self.attack * 2) # 产生1到自己攻击力的两倍之间的随机伤害
self.hp -= damage
print("{}打败了一只怪物,但是受到了{}点伤害!".format(self.name, damage))
return self.is_dead()
if __name__ == "__main__":
swordsman1 = Swordsman("张三", "剑士", 50, 100)
swordsman2 = Swordsman("李四", "剑士", 60, 120)
mage1 = Mage("王五", "法师", 80, 80)
mage2 = Mage("赵六", "法师", 70, 90)
hero_list = [swordsman1, swordsman2, mage1, mage2]
while True:
random.shuffle(hero_list) # 随机打乱英雄的顺序
for i in range(len(hero_list)):
if hero_list[i].is_dead(): # 如果英雄已经死亡,跳过这个回合
continue
print("轮到{}出手了!".format(hero_list[i].name))
action = input("请选择攻击方式(1.物理攻击 2.防御 3.魔法攻击 4.吃 5.打怪):")
if action == "1":
target_index = int(input("请选择攻击的目标(输入目标编号,从1开始):")) - 1
while hero_list[target_index].is_dead(): # 如果目标英雄已经死亡,重新选择目标
target_index = int(input("目标已经死亡,请重新选择攻击的目标(输入目标编号,从1开始):")) - 1
hero_list[i].attack_fight(hero_list[target_index])
elif action == "2":
target_index = int(input("请选择攻击的目标(输入目标编号,从1开始):")) - 1
while hero_list[target_index].is_dead(): # 如果目标英雄已经死亡,重新选择目标
target_index = int(input("目标已经死亡,请重新选择攻击的目标(输入目标编号,从1开始):")) - 1
hero_list[i].defense_fight(hero_list[target_index])
elif action == "3":
target_index = int(input("请选择攻击的目标(输入目标编号,从1开始):")) - 1
while hero_list[target_index].is_dead(): # 如果目标英雄已经死亡,重新选择目标
target_index = int(input("目标已经死亡,请重新选择攻击的目标(输入目标编号,从1开始):")) - 1
hero_list[i].magic_fight(hero_list[target_index])
elif action == "4":
hero_list[i].eat()
elif action == "5":
hero_list[i].fight_with_monster()
else:
print("无效的操作!请重新选择。")
continue
# 英雄死亡后从列表中移除
for hero in hero_list:
if hero.is_dead():
hero_list.remove(hero)
# 判断是否只剩下一个英雄
if len(hero_list) == 1:
print("游戏结束,{}获胜!".format(hero_list[0].name))
exit()
```
在这个程序中,我们定义了一个基类 `Hero`,并且派生出了两个子类 `Swordsman` 和 `Mage`。每个英雄对象都有名字、类型、攻击力和生命值等属性,并且实现了不同的战斗技能和增加生命值的方法。
在主函数中,我们创建了四个不同的英雄对象,并将它们添加到一个列表中。然后,我们进入游戏循环,随机打乱英雄的顺序,让每个英雄依次出手。在每个回合中,我们让玩家选择攻击方式和攻击目标,并且根据不同的攻击方式计算伤害。如果英雄的生命值小于等于0,表示已经被对方杀死,从列表中移除。当只剩下一个英雄时,游戏结束,输出获胜者的名字。
阅读全文
相关推荐


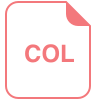
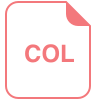
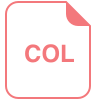
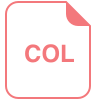
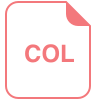
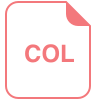

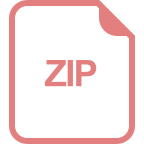
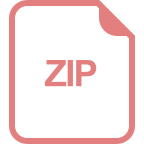
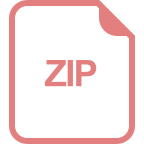
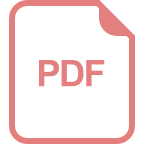