用cavas写一个樱花飘落页面
时间: 2024-03-20 16:40:56 浏览: 22
好的,以下是一个使用Canvas实现樱花飘落的页面,代码中有注释解释每个部分的作用:
```html
<!DOCTYPE html>
<html>
<head>
<title>樱花飘落页面</title>
<style>
body {
margin: 0;
padding: 0;
background-color: #f9d2e9;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script>
// 获取canvas元素
var canvas = document.getElementById("canvas");
// 设置canvas大小为屏幕大小
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 获取画布上下文
var ctx = canvas.getContext("2d");
// 定义樱花花瓣数组
var sakuras = [];
// 定义樱花花瓣类
function Sakura(x, y, r, vx, vy) {
this.x = x;
this.y = y;
this.r = r;
this.vx = vx;
this.vy = vy;
this.alpha = 1;
this.color = "#FFC0CB";
}
// 定义樱花花瓣渲染方法
Sakura.prototype.render = function() {
ctx.beginPath();
ctx.globalAlpha = this.alpha;
ctx.fillStyle = this.color;
ctx.arc(this.x, this.y, this.r, 0, Math.PI * 2);
ctx.fill();
}
// 定义樱花花瓣更新方法
Sakura.prototype.update = function() {
// 计算新位置
this.x += this.vx;
this.y += this.vy;
// 计算新透明度
this.alpha -= 0.01;
// 如果透明度小于等于0,就从数组中删除
if (this.alpha <= 0) {
sakuras.splice(sakuras.indexOf(this), 1);
}
}
// 定义樱花花瓣生成函数
function createSakura() {
// 随机生成樱花花瓣的位置、大小和速度
var x = Math.random() * canvas.width;
var y = -50;
var r = Math.random() * 10 + 10;
var vx = Math.random() * 2 - 1;
var vy = Math.random() * 2 + 1;
// 创建新的樱花花瓣对象并添加到数组中
var sakura = new Sakura(x, y, r, vx, vy);
sakuras.push(sakura);
}
// 定义动画函数
function animate() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 每隔一段时间生成新的樱花花瓣
if (Math.random() > 0.9) {
createSakura();
}
// 遍历每个樱花花瓣,更新位置并渲染
for (var i = 0; i < sakuras.length; i++) {
var sakura = sakuras[i];
sakura.update();
sakura.render();
}
// 循环调用动画函数
requestAnimationFrame(animate);
}
// 启动动画函数
animate();
</script>
</body>
</html>
```
这个页面使用Canvas实现了樱花花瓣的飘落效果,每隔一段时间会随机生成新的花瓣,并使用透明度和速度控制花瓣的位置和渲染。通过循环调用动画函数,使得花瓣会不断地飘落,营造出樱花飞舞的效果。
相关推荐
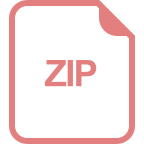
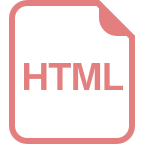











