请你就前三点用代码举例详细说明
时间: 2024-02-16 10:00:26 浏览: 71
好的,下面我将分别就前三点使用 Python 代码举例说明。
1. 数据处理
数据处理是后端开发中非常重要的一项工作,使用算法可以帮助开发者更快速、高效地处理数据。例如,下面是一个使用归并排序算法对列表进行排序的 Python 代码示例:
```python
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left_arr = merge_sort(arr[:mid])
right_arr = merge_sort(arr[mid:])
return merge(left_arr, right_arr)
def merge(left_arr, right_arr):
result = []
left_index, right_index = 0, 0
while left_index < len(left_arr) and right_index < len(right_arr):
if left_arr[left_index] < right_arr[right_index]:
result.append(left_arr[left_index])
left_index += 1
else:
result.append(right_arr[right_index])
right_index += 1
result += left_arr[left_index:]
result += right_arr[right_index:]
return result
arr = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_arr = merge_sort(arr)
print(sorted_arr)
```
这段代码是一个基于递归实现的归并排序算法,可以对任意长度的列表进行排序。通过使用该算法,我们可以高效地对大量数据进行排序。
2. 优化性能
性能优化是后端开发中非常重要的一项工作,使用算法可以帮助开发者优化服务的性能。例如,下面是一个使用哈希表实现缓存功能的 Python 代码示例:
```python
class LRUCache:
def __init__(self, capacity):
self.cache = {}
self.capacity = capacity
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key):
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.val
else:
return -1
def put(self, key, value):
if key in self.cache:
node = self.cache[key]
self._remove(node)
node.val = value
self._add(node)
else:
if len(self.cache) == self.capacity:
node = self.head.next
self._remove(node)
del self.cache[node.key]
node = Node(key, value)
self.cache[key] = node
self._add(node)
def _remove(self, node):
prev = node.prev
next = node.next
prev.next = next
next.prev = prev
def _add(self, node):
prev = self.tail.prev
prev.next = node
node.prev = prev
node.next = self.tail
self.tail.prev = node
class Node:
def __init__(self, key, val):
self.key = key
self.val = val
self.prev = None
self.next = None
```
这段代码是一个基于哈希表和双向链表实现的 LRU 缓存功能,可以高效地保存最近使用的数据,并在缓存满时删除最久未使用的数据。通过使用该算法,我们可以优化服务的响应时间和资源开销。
3. 业务逻辑
业务逻辑是后端开发中最为重要的一项工作,使用算法可以帮助开发者更好地设计出解决方案。例如,下面是一个使用 Dijkstra 算法计算最短路径的 Python 代码示例:
```python
import heapq
def dijkstra(graph, start):
distances = {node: float('inf') for node in graph}
distances[start] = 0
queue = [(0, start)]
while queue:
current_distance, current_node = heapq.heappop(queue)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(queue, (distance, neighbor))
return distances
graph = {
'A': {'B': 10, 'C': 3},
'B': {'C': 1, 'D': 2},
'C': {'B': 4, 'D': 8, 'E': 2},
'D': {'E': 7},
'E': {'D': 9}
}
distances = dijkstra(graph, 'A')
print(distances)
```
这段代码是一个基于 Dijkstra 算法实现的最短路径计算功能,可以计算任意两点之间的最短路径。通过使用该算法,我们可以更好地设计出解决方案,实现各种业务需求。
阅读全文
相关推荐




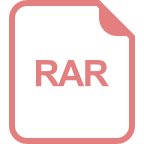



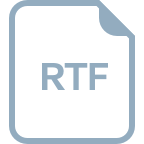

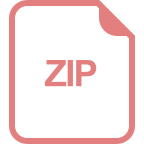



