MultipartFile获取文件上传路径
时间: 2023-10-14 11:27:18 浏览: 678
MultipartFile并不是用来获取文件上传路径的,它是用来接收上传文件的。要获取上传文件的路径,可以通过HttpServletRequest获取。示例如下:
```java
@RequestMapping(value = "/upload", method = RequestMethod.POST)
public String upload(@RequestParam("file") MultipartFile file, HttpServletRequest request) {
String uploadPath = request.getSession().getServletContext().getRealPath("/") + "upload/";
// ...
}
```
这里用到了HttpServletRequest的getSession()方法和getServletContext()方法来获取ServletContext对象,再通过getRealPath()方法获取上传文件的路径。注意要在web.xml中配置上传文件存储的路径,否则会抛出空指针异常。
相关问题
multipartfile获取文件绝对路径
在Spring框架中,MultipartFile是用于处理上传文件的接口,它提供了一系列用于获取文件信息的方法,但是没有直接提供获取文件绝对路径的方法。
这是因为在Web应用中,文件上传时直接存储到服务器的临时目录中,并不会将文件保存在指定的绝对路径下。这样设计的目的是为了安全性考虑,防止直接将文件保存到应用程序目录或其他敏感目录中。
如果需要获取MultipartFile对象对应文件的绝对路径,我们可以通过以下方式实现:
1. 获取ServletContext对象:可以在Spring MVC的Controller中通过注入HttpServletRequest对象,然后调用`request.getServletContext()`方法获取。
2. 获取MultipartFile的InputStream对象:通过`multipartFile.getInputStream()`方法获取。
3. 创建ServletOutputStream对象:可以通过ServletContext的`getRealPath()`方法获取文件所在的绝对路径,然后利用该绝对路径创建文件输出流对象。
以下是示例代码:
```java
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import java.io.*;
@RestController
public class FileController {
@PostMapping("/upload")
public String uploadFile(@RequestParam("file") MultipartFile file, HttpServletRequest request) {
try {
ServletContext context = request.getServletContext();
InputStream input = file.getInputStream();
String realPath = context.getRealPath("/");
String filePath = realPath + "uploads/" + file.getOriginalFilename();
OutputStream output = new FileOutputStream(new File(filePath));
int bytesRead;
byte[] buffer = new byte[8192];
while ((bytesRead = input.read(buffer, 0, 8192)) != -1) {
output.write(buffer, 0, bytesRead);
}
input.close();
output.close();
return "文件上传成功,保存路径为:" + filePath;
} catch (IOException ex) {
ex.printStackTrace();
return "文件上传失败!";
}
}
}
```
通过上述代码,我们可以将上传的文件保存到服务器指定的绝对路径下,并且返回文件的绝对路径信息。
multipartfile获取文件路径
关于multipartfile获取文件路径的问题,我们可以通过如下方式获取:
1. 首先,在控制层(Controller)中定义一个multipartfile类型的参数来接收上传的文件,例如:
```
@PostMapping("/uploadFile")
public String uploadFile(@RequestParam("file") MultipartFile file) {
// 处理文件上传操作
}
```
2. 然后,在方法体内,我们可以通过file.getOriginalFilename()方法获取上传文件的原始文件名,例如:
```
String fileName = file.getOriginalFilename();
```
3. 接下来,我们可以通过System.getProperty("user.dir")方法获取当前项目的根目录路径,例如:
```
String rootPath = System.getProperty("user.dir");
```
4. 最后,我们可以将上传文件保存在项目根目录下的一个指定文件夹中,例如:
```
String savePath = rootPath + "/uploadFiles/";
File fileDirectory = new File(savePath);
if (!fileDirectory.exists()) {
fileDirectory.mkdirs();
}
File saveFile = new File(savePath + fileName);
try {
file.transferTo(saveFile);
return "文件上传成功!";
} catch (IOException e) {
return "文件上传失败!";
}
```
通过以上方法,我们可以获取上传文件的文件路径,并将文件保存在指定位置中。希望这能够帮到你。
阅读全文
相关推荐
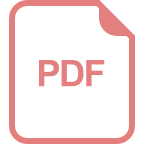













