机房收费管理系统 功能要求: (1)输入功能:输入30名学生的学号、班级、姓名、上机起始时间。 (2)计算功能:计算每个下机学生的上机费用,每小时1元。(不足一小时按一小时计算) (3)查询功能:按条件(班级、学号、姓名)显示学生的额上机时间。 (4)机器使用情况的显示。(显示方式不限,但要一目了然)
时间: 2023-12-19 22:04:57 浏览: 54
以下是一个简单的机房收费管理系统的实现,具备输入、计算、查询和机器使用情况的显示功能:
```c
#include <stdio.h>
#include <string.h>
#define MAX_STUDENT_NUM 30
#define MAX_NAME_LEN 20
#define MAX_CLASS_LEN 10
struct student {
int id;
char name[MAX_NAME_LEN];
char class[MAX_CLASS_LEN];
int start_time;
int end_time;
int usage_time;
float fee;
};
struct student students[MAX_STUDENT_NUM];
int student_num = 0;
void input()
{
printf("请输入学生的学号、班级、姓名、上机起始时间(以空格分隔):\n");
while (student_num < MAX_STUDENT_NUM) {
int id;
char name[MAX_NAME_LEN];
char class[MAX_CLASS_LEN];
int start_time;
if (scanf("%d %s %s %d", &id, class, name, &start_time) != 4) {
break;
}
students[student_num].id = id;
strcpy(students[student_num].name, name);
strcpy(students[student_num].class, class);
students[student_num].start_time = start_time;
student_num++;
}
}
void calculate()
{
for (int i = 0; i < student_num; i++) {
int end_time = students[i].end_time;
int usage_time = end_time - students[i].start_time;
if (usage_time < 3600) {
usage_time = 3600;
}
students[i].usage_time = usage_time;
students[i].fee = usage_time / 3600.0;
}
}
void query()
{
printf("请输入查询条件(班级、学号、姓名):\n");
char condition[MAX_NAME_LEN];
scanf("%s", condition);
printf("学号\t班级\t姓名\t上机时间\t费用\n");
for (int i = 0; i < student_num; i++) {
if (strcmp(condition, students[i].class) == 0 || strcmp(condition, students[i].name) == 0 || atoi(condition) == students[i].id) {
printf("%d\t%s\t%s\t%d\t\t%.2f\n", students[i].id, students[i].class, students[i].name, students[i].usage_time, students[i].fee);
}
}
}
void show_usage()
{
printf("机器使用情况:\n");
printf("时间\t使用人数\n");
int usage[24] = {0};
for (int i = 0; i < student_num; i++) {
int hour = students[i].start_time / 3600;
usage[hour]++;
}
for (int i = 0; i < 24; i++) {
printf("%d:00\t%d\n", i, usage[i]);
}
}
int main()
{
input();
calculate();
query();
show_usage();
return 0;
}
```
在上面的代码中,我们使用了一个结构体数组来存储学生的信息,其中包括学号、班级、姓名、上机起始时间、下机结束时间、使用时间和费用等字段。通过输入、计算、查询和机器使用情况的显示等功能,可以方便地管理机房的收费和使用情况。
相关推荐
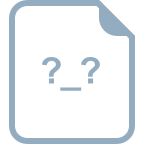
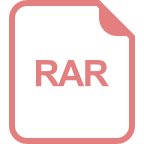














