canny c++ 实现
时间: 2023-08-20 11:04:00 浏览: 84
Canny算法是一种边缘检测算法,可以在图像上找到边缘。以下是C++实现的基本步骤:
1. 对图像进行灰度化处理。
2. 对灰度图像进行高斯滤波,以去除噪声。
3. 计算图像中每个像素的梯度幅值和方向。
4. 对梯度方向进行非极大值抑制,以保留边缘的细节。
5. 应用双阈值算法,将像素分类为强边缘、弱边缘和非边缘。
6. 进行边缘连接,以连接强边缘和相邻的弱边缘。
下面是C++实现的示例代码,以OpenCV库为例:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main(int argc, char** argv)
{
Mat src, src_gray, dst;
int edgeThresh = 1;
int lowThreshold, highThreshold;
int const max_lowThreshold = 100;
int const max_highThreshold = 300;
int const kernel_size = 3;
char* window_name = "Edge Map";
src = imread(argv[1], IMREAD_COLOR);
if (src.empty())
{
cout << "Could not open or find the image!\n" << endl;
return -1;
}
cvtColor(src, src_gray, COLOR_BGR2GRAY);
blur(src_gray, dst, Size(3, 3));
Canny(dst, dst, lowThreshold, highThreshold, kernel_size);
dst = Scalar::all(0);
src.copyTo(dst, dst);
imshow(window_name, dst);
waitKey(0);
return 0;
}
```
在这个示例中,我们使用了OpenCV中的Canny函数来实现Canny算法。首先,我们将图像转换为灰度图像,然后进行高斯滤波。接下来,我们使用Canny函数计算边缘,并将结果显示在窗口中。
阅读全文
相关推荐
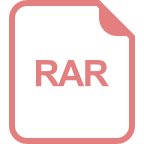
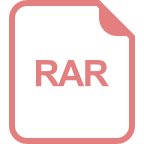
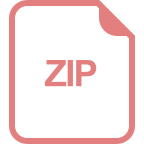
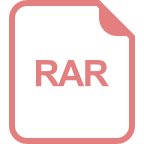
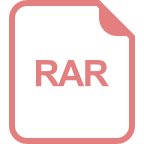
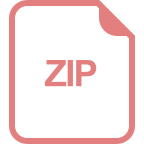
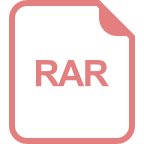
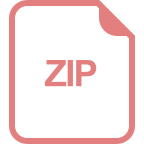
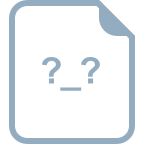
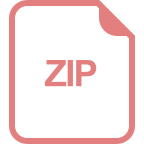
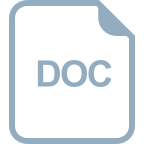
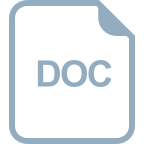



